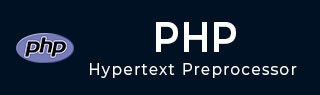
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - Returning Values
A PHP function can have an optional return statement as the last statement in its function body. Most of the built-in functions in PHP return a certain value. For example the strlen() function returns the length of a string. Similarly, a user defined function can also return a certain value.
A function is an independent, complete and reusable block of statements. When called, it performs a certain task and sends the program control back to position from where it was called even if return statement is not used. The return statement allows it to take a value, along with the control, back to the calling environment.
function foo($arg_1, $arg_2) { statements; return $retval; }
A function may return any type of data, including scalar variables, arrays and objects. A return keyword without any expression in front of it returns null, and is equivalent to a function not having a return value at all.
The value returned by a function can be stored in a variable, can be put in an expression, or, if appearing inside print or echo, is displayed in the output.
$res = foo($x, $y);
It allows the returned value of a function to be used further in the program.
Example
Let us modify the addition() function in the previous chapter to include a return statement to return the result of addition.
<?php function addition($first, $second) { $result = $first+$second; return $result; } $x=10; $y=20; $z = addition($x, $y); echo "First number: $x Second number: $y Addition: $z". PHP_EOL; ?>
It will produce the following output −
First number: 10 Second number: 20 Addition: 30
A function in PHP may have any number of arguments, but can return only one value. The function goes back to the calling environment as soon as it comes across a return statement for the first time, abandoning the rest of statements in the function body.
Example
If you try to include more than one values in the return statement, a PHP parse error is encountered as below −
<?php function raiseto($x) { $sqr = $x**2; $cub = $x**3; return $sqr, $cub; } $a = 5; $val = raiseto($a); ?>
It will produce the following output −
PHP Parse error: syntax error, unexpected token ",", expecting ";"
Conditional Return
You can have multiple return statements executed under different conditional statements.
Example
In the following program, the raiseto() function returns either the square or the cube of a number of the index argument, that is either 2 or 3, respectively.
<?php function raiseto($x, $i) { if ($i == 2) { return $x**2; } elseif ($i==3) { return $x**3; } } $a = 5; $b = 2; $val = raiseto($a, $b); echo "$a raised to $b = $val" . PHP_EOL; $x = 7; $y = 3; echo "$x raised to $y = " . raiseto($x, $y) . PHP_EOL; ?>
It will produce the following output −
5 raised to 2 = 25 7 raised to 3 = 343
Return Multiple Values as Array
The function in PHP is capable of returning only a single value. However, that single value can be an array of more than one values. We can take the advantage of this feature to return the square as well as the cube of a number at once.
Example
Take a look at the following example −
<?php function raiseto($x){ $sqr = $x**2; $cub = $x**3; $ret = ["sqr" => $sqr, "cub" => $cub]; return $ret; } $a = 5; $val = raiseto($a); echo "Square of $a: " . $val["sqr"] . PHP_EOL; echo "Cube of $a: " . $val["cub"] . PHP_EOL; ?>
It will produce the following output −
Square of 5: 25 Cube of 5: 125