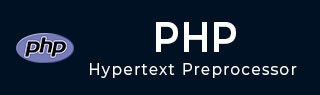
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP – IntlChar
In PHP7, a new IntlChar class has been introduced. It provides access to a number of utility methods that can be used to access information about Unicode characters. There are a number of static methods and constants in Intl class. They adhere closely to the names and behavior used by the underlying ICU (International Components for Unicode) library.
Note that you need to enable the Intl extension in the PHP installation in your system. To enable, open php.ini file and uncomment (remove the leading semicolon from the line)
extension=intl
Some static functions from Intl class are explained with examples as below −
IntlChar::charAge
This function gets the "age" of the code point
public static IntlChar::charAge(int|string $codepoint): ?array
The "age" is the Unicode version when the code point was first designated (as a non-character or for Private Use) or assigned a character.
Example
Take a look at the following example −
<?php var_dump(IntlChar::charage("\u{2603}")); ?>
It will produce the following output −
array(4) { [0]=> int(1) [1]=> int(1) [2]=> int(0) [3]=> int(0) }
IntlChar::charFromName
The charFromName() function finds Unicode character by name and return its code point value
public static IntlChar::charFromName(string $name, int $type = IntlChar::UNICODE_CHAR_NAME): ?int
The type parameter sets of names to use for the lookup. Can be any of these constants −
IntlChar::UNICODE_CHAR_NAME (default)
IntlChar::UNICODE_10_CHAR_NAME
IntlChar::EXTENDED_CHAR_NAME
IntlChar::CHAR_NAME_ALIAS
IntlChar::CHAR_NAME_CHOICE_COUNT
Example
Take a look at the following example −
<?php var_dump(IntlChar::charFromName("LATIN CAPITAL LETTER A")); var_dump(IntlChar::charFromName("SNOWMAN")); ?>
It will produce the following output −
int(65) int(9731)
IntlChar::charName
The charName() function retrieves the name of a Unicode character
public static IntlChar::charName(int|string $codepoint, int $type = IntlChar::UNICODE_CHAR_NAME): ?string
Example
Take a look at the following example −
<?php var_dump(IntlChar::charName(".", IntlChar::UNICODE_CHAR_NAME)); var_dump(IntlChar::charName("\u{2603}")); ?>
It will produce the following output −
string(9) "FULL STOP" string(7) "SNOWMAN"
IntlChar::isalpha
The isalpha() function determines whether the specified code point is a letter character. true for general categories "L" (letters).
public static IntlChar::isalpha(int|string $codepoint): ?bool
Example
Take a look at the following example −
<?php var_dump(IntlChar::isalpha("A")); var_dump(IntlChar::isalpha("1")); ?>
It will produce the following output −
bool(true) bool(false)
The Intl class defines similar static methods such as isdigit(), isalnum(), isblank(), etc.
IntlChar::islower
The islower() function determines whether the specified code point has the general category "Ll" (lowercase letter).
public static IntlChar::islower(int|string $codepoint): ?bool
Example
Take a look at the following example −
<?php var_dump(IntlChar::islower("A")); var_dump(IntlChar::islower("a")); ?>
It will produce the following output −
bool(false) bool(true)
Similarly, there are functions such as isupper(), istitle(), iswhitespace() etc.
IntlChar::toupper
The given character is mapped to its uppercase equivalent.
public static IntlChar::toupper(int|string $codepoint): int|string|null
If the character has no uppercase equivalent, the character itself is returned.
Example
Take a look at the following example −
<?php var_dump(IntlChar::toupper("A")); var_dump(IntlChar::toupper("a")); ?>
It will produce the following output −
string(1) "A" string(1) "A"