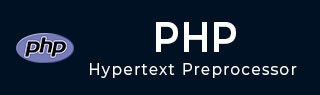
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
PHP - Switch Statement
The switch statement in PHP can be treated as an alternative to a series of if…else statements on the same expression. Suppose you need to compare an expression or a variable with many different values and execute a different piece of code depending on which value it equals to. In such a case, you would use multiple if…elseif…else constructs.
However, such a construct can make the code quite messy and difficult to follow. To simplify such codes, you can use the switch case construct in PHP that offers a more compact alternative to avoid long blocks of if..elseif..else codes.
The following PHP script uses if elseif statements −
if ($x == 0) { echo "x equals 0"; } elseif ($x == 1) { echo "i equals 1"; } elseif ($x == 2) { echo "x equals 2"; }
You can get the same result by using the switch case statements as shown below −
switch ($x) { case 0: echo "x equals 0"; break; case 1: echo "x equals 1"; break; case 2: echo "x equals 2"; break; }
The switch statement is followed by an expression, which is successively compared with value in each case clause. If it is found that the expression matches with any of the cases, the corresponding block of statements is executed.
The switch statement executes the statements inside the curly brackets line by line.
If and when a case statement is found whose expression evaluates to a value that matches the value of the switch expression, PHP starts to execute the statements until the end of the switch block, or the first time it encounters a break statement.
If you don't write a break statement at the end of a case's statement list, PHP will go on executing the statements of the following case.
Example
Try to run the above code by removing the breaks. If the value of x is 0, you’ll find that the output includes "x equals 1" as well as "x equals 2" lines.
<?php $x=0; switch ($x) { case 0: echo "x equals 0 \n"; case 1: echo "x equals 1 \n"; case 2: echo "x equals 2"; } ?>
It will produce the following output −
x equals 0 x equals 1 x equals 2
Thus, it is important make sure to end each case block with a break statement.
The Default Case in Switch
A special case is the default case. This case matches anything that wasn't matched by the other cases. Using default is optional, but if used, it must be the last case inside the curly brackets.
You can club more than one cases to simulate multiple logical expressions combined with the or operator.
<?php $x=10; switch ($x) { case 0: case 1: case 2: echo "x between 0 and 2 \n"; break; default: echo "x is less than 0 or greater than 2"; } ?>
The values to be compared against are given in the case clause. The value can be a number, a string, or even a function. However you cannot use comparison operators (<, > == or !=) as a value in the case clause.
You can choose to use semicolon instead of colon in the case clause. If no matching case found, and there is no default branch either, then no code will be executed, just as if no if statement was true.
The switch-endswitch Statement
PHP allows the usage of alternative syntax by delimiting the switch construct with switch-endswitch statements. The following version of switch case is acceptable.
<?php $x=0; switch ($x) : case 0: echo "x equals 0"; break; case 1: echo "x equals 1 \n"; break; case 2: echo "x equals 2 \n"; break; default: echo "None of the above"; endswitch ?>
Using the Break Statement in Switch…Case
Obviously, you needn’t write a break to terminate the default case, it being the last case in the switch construct.
Example
Take a look at the following example −
<?php $d = date("D"); switch ($d){ case "Mon": echo "Today is Monday"; break; case "Tue": echo "Today is Tuesday"; break; case "Wed": echo "Today is Wednesday"; break; case "Thu": echo "Today is Thursday"; break; case "Fri": echo "Today is Friday"; break; case "Sat": echo "Today is Saturday"; break; case "Sun": echo "Today is Sunday"; break; default: echo "Wonder which day is this ?"; } ?>
It will produce the following output −
Today is Monday