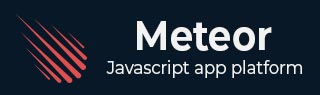
- Meteor Tutorial
- Meteor - Home
- Meteor - Overview
- Meteor - Environment Setup
- Meteor - First Application
- Meteor - Templates
- Meteor - Collections
- Meteor - Forms
- Meteor - Events
- Meteor - Session
- Meteor - Tracker
- Meteor - Packages
- Meteor - Core API
- Meteor - Check
- Meteor - Blaze
- Meteor - Timers
- Meteor - EJSON
- Meteor - HTTP
- Meteor - Email
- Meteor - Assets
- Meteor - Security
- Meteor - Sorting
- Meteor - Accounts
- Meteor - Methods
- Meteor - Package.js
- Meteor - Publish & Subscribe
- Meteor - Structure
- Meteor - Deployment
- Meteor - Running on mobile
- Meteor - ToDo App
- Meteor - Best Practices
- Meteor Useful Resources
- Meteor - Quick Guide
- Meteor - Useful Resources
- Meteor - Discussion
Meteor - Collections
In this chapter, we will learn how to use MongoDB collections.
Create a Collection
We can create a new collection with the following code −
meteorApp.js
MyCollection = new Mongo.Collection('myCollection');
Add Data
Once the collection is created, we can add data by using the insert method.
meteorApp.js
MyCollection = new Mongo.Collection('myCollection'); var myData = { key1: "value 1...", key2: "value 2...", key3: "value 3...", key4: "value 4...", key5: "value 5..." } MyCollection.insert(myData);
Find Data
We can use the find method to search for data in the collection.
meteorApp.js
MyCollection = new Mongo.Collection('myCollection'); var myData = { key1: "value 1...", key2: "value 2...", key3: "value 3...", key4: "value 4...", key5: "value 5..." } MyCollection.insert(myData); var findCollection = MyCollection.find().fetch(); console.log(findCollection);
The console will show the data we inserted previously.
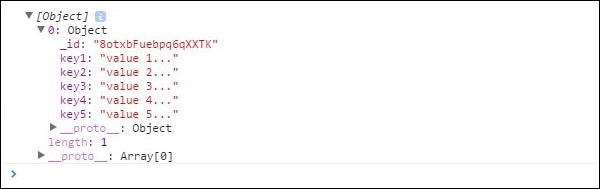
We can get the same result by adding the search parameters.
meteorApp.js
MyCollection = new Mongo.Collection('myCollection'); var myData = { key1: "value 1...", key2: "value 2...", key3: "value 3...", key4: "value 4...", key5: "value 5..." } MyCollection.insert(myData); var findCollection = MyCollection.find({key1: "value 1..."}).fetch(); console.log(findCollection);
Update Data
The next step is to update our data. After we have created a collection and inserted new data, we can use the update method.
meteorApp.js
MyCollection = new Mongo.Collection('myCollection'); var myData = { key1: "value 1...", key2: "value 2...", key3: "value 3...", key4: "value 4...", key5: "value 5..." } MyCollection.insert(myData); var findCollection = MyCollection.find().fetch(); var myId = findCollection[0]._id; var updatedData = { key1: "updated value 1...", key2: "updated value 2...", key3: "updated value 3...", key4: "updated value 4...", key5: "updated value 5..." } MyCollection.update(myId, updatedData); var findUpdatedCollection = MyCollection.find().fetch(); console.log(findUpdatedCollection);
The console will show that our collection is updated.
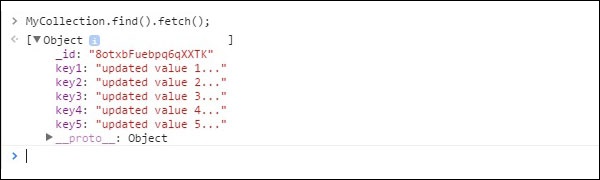
Delete Data
Data can be deleted from the collection using the remove method. We are setting id in this example as a parameter to delete specific data.
meteorApp.js
MyCollection = new Mongo.Collection('myCollection'); var myData = { key1: "value 1...", key2: "value 2...", key3: "value 3...", key4: "value 4...", key5: "value 5..." } MyCollection.insert(myData); var findCollection = MyCollection.find().fetch(); var myId = findCollection[0]._id; MyCollection.remove(myId); var findDeletedCollection = MyCollection.find().fetch(); console.log(findDeletedCollection);
The console will show an empty array.

If we want to delete everything from the collection, we can use the same method, however, instead of id we will use an empty object {}. We need to do this on the server for security reasons.
meteorApp.js
if (Meteor.isServer) { MyCollection = new Mongo.Collection('myCollection'); var myData = { key1: "value 1...", key2: "value 2...", key3: "value 3...", key4: "value 4...", key5: "value 5..." } MyCollection.insert(myData); MyCollection.remove({}); var findDeletedCollection = MyCollection.find().fetch(); console.log(findDeletedCollection); }
We can also delete data using other parameters. As in the previous example, Meteor will force us to do this from the server.
meteorApp.js
if (Meteor.isServer) { MyCollection = new Mongo.Collection('myCollection'); var myData = { key1: "value 1...", key2: "value 2...", key3: "value 3...", key4: "value 4...", key5: "value 5..." } MyCollection.insert(myData); MyCollection.remove({key1: "value 1..."}); var findDeletedCollection = MyCollection.find().fetch(); console.log(findDeletedCollection); }
It can be seen that the data is deleted from the command window.
