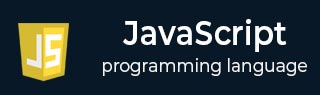
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Syntax
JavaScript Syntax
JavaScript syntax comprises a set of rules that define how to construct a JavaScript code. JavaScript can be implemented using JavaScript statements that are placed within the <script>... </script> HTML tags in a web page.
You can place the <script> tags, containing your JavaScript, anywhere within your web page, but it is normally recommended that you should keep it within the <head> tags.
The <script> tag alerts the browser program to start interpreting all the text between these tags as a script. A simple syntax of your JavaScript will appear as follows.
<script ...> JavaScript code </script>The script tag takes two important attributes −
Language −This attribute specifies what scripting language you are using. Typically, its value will be javascript. Although recent versions of HTML (and XHTML, its successor) have phased out the use of this attribute.
Type −This attribute is what is now recommended to indicate the scripting language in use and its value should be set to "text/javascript". JavaScript has become default lannguage in HTML5, and modern browsers, so now adding type is not required.
So your JavaScript segment will look like −
<script language = "javascript" type = "text/javascript"> JavaScript code </script>
Your First JavaScript Code
Let us take a sample example to print out "Hello World". We call document.write method which writes a string into our HTML document. This method can be used to write text, HTML, or both. Take a look at the following code −
<html> <head> <title> Your first JavaScript program </title> <head> <body> <script language = "javascript" type = "text/javascript"> document.write("Hello World!") </script> </body> </html>
This code will produce the following result −
Hello World!
JavaScript Values
In JavaScript, you can have two types of values.
Fixed values (Literals)
Variables (Dynamic values)
JavaScript Literals
In the below code, 10 is a Number literal and ‘Hello’ is a string literal.
<html> <body> <script> document.write(10); // Number Literal document.write("<br />"); // To add line-break document.write("Hello"); // String Literal </script> </body> </html>
This code will produce the following result −
10 Hello
JavaScript Variables
In JavaScript, variables are used to store the dynamic data.
You can use the below keyword to define variables in JavaScript.
- var
- let
- const
You can use the assignment operator (equal sign) to assign values to the variable.
In the below code, variable a contains the numeric value, and variable b contains the text (string).
<html> <body> <script> let a = 5; // Variable Declaration document.write(a); // Using variable document.write("<br>"); let b = "One"; document.write(b); </script> </body> </html>
This code will produce the following result −
5 One
Whitespace and Line Breaks
JavaScript ignores spaces, tabs, and newlines that appear in JavaScript programs. You can use spaces, tabs, and newlines freely in your program and you are free to format and indent your programs in a neat and consistent way that makes the code easy to read and understand.
Semicolons are Optional
Simple statements in JavaScript are generally followed by a semicolon character, just as they are in C, C++, and Java. JavaScript, however, allows you to omit this semicolon if each of your statements are placed on a separate line. For example, the following code could be written without semicolons.
<script> var1 = 10 var2 = 20 </script>
But when formatted in a single line as follows, you must use semicolons −
<script> var1 = 10; var2 = 20; </script>
It is a good programming practice to use semicolons.
Case Sensitivity
JavaScript is a case-sensitive language. This means that the language keywords, variables, function names, and any other identifiers must always be typed with a consistent capitalization of letters.
So the identifiers Time and TIME will convey different meanings in JavaScript.
In the code below, we compare the ‘time’ and ‘Time’ strings, which returns false.
<html> <body> <script> let a = "time"; let b = "Time"; document.write("a == b? " + (a == b)); </script> </body> </html>
This code will produce the following result −
a == b? false
Care should be taken while writing variable and function names in JavaScript.
JavaScript and Camel Case
Pascal Case − We can create variables like SmartWatch, MobileDevice, WebDrive, etc. It represents the upper camel case string.
Lower Camel Case − JavaScript allows developers to use variable names and expression names like smartwatch, mobileDevice, webDriver, etc. Here the first character is in lowercase.
Underscore − We can use underscore while declaring variables like smart_watch, mobile_device, web_driver, etc.
JavaScript doesn’t allow adding the hyphen in variable name or expression name.
JavaScript Keywords
JavaScript contains multiple keywords which we can use for a particular task. For example, the function keyword can be used to define the function. The let, var, and const keywords can be used to define variables.
Let’s understand the use of the keyword via the example below.
Example
In this example, we used the function keyword to define the function. We used the var keyword inside the function to define the sum variable.
Also, we used the let and const keywords outside the function to define two variables and initialize them with values. After that, we called the function using the function name and passed variables as an argument.
<html> <body> <script> function getSum(first, second) { var sum = first * second; document.write("The sum of " + first + " and " + second + " is " + sum); } let first = 3; const second = 4; getSum(first, second); </script> </body> </html>
This code will produce the following result −
The sum of 3 and 4 is 12
JavaScript doesn’t allow to use the of keywords as a variable or expression name.
JavaScript Identifiers
In JavaScript, identifiers are the name of variables, functions, objects, etc.
For example, p is an identifier in the below code.
<script> pet p = 90; </script>
The 'test' is an identifier in the below code.
<script> function test() { } </script>
Here are the rules which you should follow to define valid identifiers.
Identifiers should always start with the alphabetical characters (A-Z, a-z), $(dollar sign), or _ (underscore).
It shouldn’t start with digits or hyphens.
The identifier can also contain digits except for the start of it.
Comments in JavaScript
JavaScript supports both C-style and C++-style comments, Thus −
Any text between a // and the end of a line is treated as a comment and is ignored by JavaScript.
Any text between the characters /* and */ is treated as a comment. This may span multiple lines.
JavaScript also recognizes the HTML comment opening sequence <!--. JavaScript treats this as a single-line comment, just as it does the // comment.
The HTML comment closing sequence --> is not recognized by JavaScript so it should be written as //-->.
Example
The following example shows how to use comments in JavaScript.
<script> // This is a comment. It is similar to comments in C++ /* * This is a multi-line comment in JavaScript * It is very similar to comments in C Programming */ </script>
Operators in JavaScript
JavaScript contains various arithmetic, logical, bitwise, etc. operators. We can use any operator in JavaScript, as shown in the example below.
Example
In this example, we have defined var1 and va2 and initialized them with number values. After that, we use the ‘*’ operator to get the multiplication result of var1 and var2.
<html> <body> <script> var1 = 10 var2 = 20 var3 = var1 * var2; var4 = 10 + 20; document.write(var3, " " ,var4); </script> </body> </html>
This code will produce the following result −
200 30
In this way, programmers can use other operators with operands.
When any of two operands of the ‘+’ operator is a string, it converts the other operand to a string and merges both strings.
Expressions in JavaScript
You can create expressions in JavaScript by combining the variable, values, and operators.
For example, the below expression adds two numbers.
10 + 20;
The below expression multiplies the values of two variables.
a * b;
The below expression divides the value of variable c with 2.
c / 2;
Example
In the below code, we have used the assignment and arithmetic expressions.
<html> <body> <script> let a = 10; let b = 2; let c = a; // Assigning a value of a to c. Assignment expression. let d = a + b; // Adding a and b. Arithmetic expression. let e = a - b; // Subtracting b from a. document.write("c = " + c + "<br>"); document.write("d = " + d + "<br>"); document.write("e = " + e + "<br>"); </script> </body> </html>
This code will produce the following result −
c = 10 d = 12 e = 8
We will explore more expressions in the upcoming chapters.
JavaScript Character Set
JavaScript contains a set of Unicode characters.
The Unicode characters add special characters like emoji, symbols, etc. in the text.
For example, the below Unicode character will display the left arrow.
&larr
The Below Unicode character will display the RS (Rupees sign) symbol.
8360
However, there are a lot of syntaxes of JavaScript we need to cover, and it is not possible to cover them in a single chapter. So, we have covered only basic syntax in this chapter to get started with JavaScript, and we will cover other syntaxes in upcoming chapters.