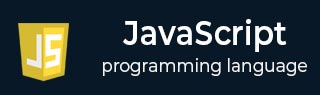
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Canvas
Handling Canvas with JavaScript
The HTML <canvas> element can be used to create and draw graphics on the web page. It can draw various graphics or shapes, like lines, circles, etc., on the web page and animate them.
You can define <canvas> element in HTML, and after that, you need to use JavaScript to draw graphics on the web page. Let's look at the syntaxes to define a canvas element in HTML and manipulate with JavaScript.
Syntax
Use the following syntax to create an HTML canvas element.
<canvas id="myCanvas" width="480" height="320"> Your browser does not support the HTML canvas tag. </canvas>
In the above syntax, we have defined the HTML <canvas> element with id as 'myCanvas'. The message written in between <canvas> tags will be displayed when the browser doesn't support the <canvas> element.
We access the canvas element using the getElementById() method of document object. Look at below syntax −
var canvas = document.getElementById('canvas_id'); var ctx = canvas.getContext('2d');
In JavaScript, we can use the getContext('2d') method to get the context of the 2D canvas. Next, we can use various methods to draw a shape and fill in the color by taking the 'ctx' variable as a reference.
Examples
Example: Drawing a rectangle
In the code below, we have added the <canvas> element in HTML.
In JavaScript, we have used the getContext('2d') method to access the context of the canvas. After that, we used the fillstyle() method to color the element in the canvas. The fillRect() method is used to draw a rectangle. It takes the coordinates of 4 corners in the canvas.
<html> <body> <h2> JavaScript - Canvas </h2> <p> Drawing Rectangle on Canvas using JavaScript</p> <canvas id = "myCanvas" width = "600" height = "300" style = "border:1px solid #000000;"> Your browser does not support the HTML canvas tag. </canvas> <script> var canvas = document.getElementById("myCanvas"); // Getting the 2D context of the canvas var ctx = canvas.getContext("2d"); // Drawing rectangle using canvas ctx.fillStyle = "#FF0000"; ctx.fillRect(50,30, 470, 240); </script> </body> </html>
Example: Drawing a circle
In the code below, we used the arc() method to draw a circle. It takes the coordinates for the circle position as the first 2 parameters and the radius of the circle as a third parameter.
<html> <body> <h2> JavaScript - Canvas </h2> <p> Drawing circle on Canvas using JavaScript</p> <canvas id = "myCanvas" width = "600" height = "300" style = "border:1px solid #000000;"> Your browser does not support the HTML canvas tag. </canvas> <script> var c = document.getElementById("myCanvas"); // Getting the 2D context of the canvas var ctx = c.getContext("2d"); // Drawing circle using canvas ctx.beginPath(); ctx.arc(300, 150, 100, 0, 2 * Math.PI); ctx.stroke(); ctx.fillStyle = "green"; ctx.fill(); </script> </body> </html>
Example: Drawing a lines
In the code below, we have drawn the lines using the moveTo() and lineTo() methods. The moveTo() method takes the starting point of the line as a parameter, and the lineTo() method takes the ending point of the line as a parameter.
<html> <body> <h2> JavaScript - Canvas </h2> <p> Drawing lines on Canvas using JavaScript</p> <canvas id = "myCanvas" width = "600" height = "300" style = "border:1px solid #000000;"> Your browser does not support the HTML canvas tag. </canvas> <script> var canvas = document.getElementById("myCanvas"); // Getting the 2D context of the canvas var ctx = canvas.getContext("2d"); // Drawing lines using canvas ctx.moveTo(0, 0); ctx.lineTo(300, 200); ctx.stroke(); ctx.moveTo(300, 200); ctx.lineTo(400, 0); ctx.stroke(); </script> </body> </html>
Example: Drawing a Linear gradient
<html> <body> <h2> JavaScript - Canvas </h2> <p> Drawing linear gradient on Canvas using JavaScript</p> <canvas id = "myCanvas" width = "600" height = "300" style = "border:1px solid #000000;"> Your browser does not support the HTML canvas tag. </canvas> <script> var canvas = document.getElementById("myCanvas"); // Getting the 2D context of the canvas var ctx = canvas.getContext("2d"); // Drawing a linear gradient ctx.beginPath(); var grd = ctx.createLinearGradient(0, 0, 440, 0); grd.addColorStop(0, "yellow"); grd.addColorStop(1, "white"); ctx.fillStyle = grd; ctx.fillRect(10, 10, 550, 240); ctx.fill(); ctx.closePath(); </script> </body> </html>
Example: Text Rendering
In the below example, we have rendered a text message "Hello" on the canvas. For this, we have used fillText() method. The fillText() method in HTML Canvas draws a text string on a canvas element.
<html> <body> <h2> JavaScript - Canvas </h2> <p> Rendering text on Canvas using JavaScript</p> <canvas id = "myCanvas" width = "600" height = "200" style = "border:1px solid #000000;"> Your browser does not support the HTML canvas tag. </canvas> <script> const canvas = document.getElementById('myCanvas'); // Getting the 2D context of the canvas const ctx = canvas.getContext('2d'); ctx.font = '30px Arial'; ctx.fillStyle = 'red'; ctx.fillText('Hello, Canvas!', 150, 100); </script> </body> </html>
We have learned to use <canvas> elements in HTML and draw graphics by accessing elements using JavaScript. We can draw various shapes and add colors to shapes.