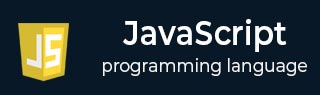
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - The Strings Object
The String object in JavaScript lets you work with a series of characters; it wraps JavaScript's string primitive data type with a number of helper methods.
As JavaScript automatically converts between string primitives and String objects, you can call any of the helper methods of the String object on a string primitive.
The string is a sequence of characters containing 0 or more characters. For example, 'Hello' is a string.
Syntax
JavaScript strings can be created as objects using the String() constructor or as primitives using string literals.
Use the following syntax to create a String object −
const val = new String(value);
The String parameter, value is a series of characters that has been properly encoded.
We can create string primitives using string literals and the String() function as follows −
str1 = 'Hello World!'; // using single quote str2 = "Hello World!"; // using double quote str3 = 'Hello World'; // using back ticks str4 = String('Hello World!'); // using String() function
String Properties
Here is a list of the properties of String object and their description.
Sr.No. | Property & Description |
---|---|
1 | constructor
Returns a reference to the String function that created the object. |
2 | length
Returns the length of the string. |
3 | prototype
The prototype property allows you to add properties and methods to an object. |
String Methods
Here is a list of the methods available in String object along with their description.
Static Methods
The static methods are invoked using the 'String' class itself.
Sr.No. | Property & Description |
---|---|
1 | fromCharCode() Converts the sequence of UTF-16 code units into the string. |
2 | fromCodePoint() Creates a string from the given sequence of ASCII values. |
Instance Methods
The instance methods are invoked using the instance of the String class.
Sr.No. | Method & Description |
---|---|
1 | at() Returns the character from the specified index. |
2 | charAt() Returns the character at the specified index. |
3 | charCodeAt()
Returns a number indicating the Unicode value of the character at the given index. |
4 | codePointAt() Returns a number indicating the Unicode value of the character at the given index. |
5 | concat()
Combines the text of two strings and returns a new string. |
6 | endsWith() Checks whether the string ends with a specific character or substring. |
7 | includes() To check whether one string exists in another string. |
8 | indexOf()
Returns the index within the calling String object of the first occurrence of the specified value, or -1 if not found. |
9 | lastIndexOf()
Returns the index within the calling String object of the last occurrence of the specified value, or -1 if not found. |
10 | localeCompare()
Returns a number indicating whether a reference string comes before or after or is the same as the given string in sort order. |
11 | match()
Used to match a regular expression against a string. |
12 | matchAll() Used to match all occurrences of regular expression patterns in the string. |
13 | normalize() To get the Unicode normalization of the string. |
14 | padEnd() To add padding to the current string with different strings at the end. |
15 | padStart() To add padding to the current string with different strings at the start. |
16 | replace()
Used to find a match between a regular expression and a string and replace the matched substring with a new one. |
17 | repalceAll() Used to find a match between a regular expression and a string and replace all the matched substring with a new one. |
18 | repeat() To get a new string containing the N number of copies of the current string. |
19 | search()
Executes the search for a match between a regular expression and a specified string. |
20 | slice()
Extracts a section of a string and returns a new string. |
21 | split()
Splits a String object into an array of strings by separating the string into substrings. |
22 | substr()
Returns the characters in a string beginning at the specified location through the specified number of characters. |
23 | substring()
Returns the characters in a string between two indexes into the string. |
24 | toLocaleLowerCase()
The characters within a string are converted to lowercase while respecting the current locale. |
25 | toLocaleUpperCase()
The characters within a string are converted to the upper case while respecting the current locale. |
26 | toLowerCase()
Returns the calling string value converted to lowercase. |
27 | toString()
Returns a string representing the specified object. |
28 | toUpperCase()
Returns the calling string value converted to uppercase. |
29 | trim() It removes white spaces from both ends. |
30 | trimEnd() It removes white spaces from the start. |
31 | trimStart() It removes white spaces from the end. |
32 | valueOf()
Returns the primitive value of the specified object. |
Example: Creating JavaScript String Objects
In the example below, we used the string() constructor with the 'new' keyword to create a string object.
<html> <head> <title> JavaScript - String Object </title> </head> <body> <p id = "output"> </p> <script> const str = new String("Hello World!"); document.getElementById("output").innerHTML = "str == " + str + "<br>" + "typeof str == " + typeof str; </script> </body> </html>
Output
str == Hello World! typeof str == object
Accessing a String
You can access the string characters using its index. The string index starts from 0.
Example
In the example below, we access the character from the 0th and 4th index of the string.
<html> <head> <title> JavaScript - Accessing a string </title> </head> <body> <p id = "output"> </p> <script> const str1 = new String("Welcome!"); let str2 = "Welcome!"; document.getElementById("output").innerHTML = "0th character is - " + str1[0] + "<br>" + "4th character is - " + str2[4]; </script> </body> </html>Output
0th character is - W 4th character is - o
JavaScript Strings are Case-sensitive
In JavaScript, strings are case-sensitive. It means lowercase and uppercase characters are different.
Example
In the example below, char1 contains the uppercase 'S', and char2 contains the lowercase 's' characters. When you compare char1 and char2, it returns false as strings are case-sensitive.
<html> <head> <title> JavaScript - String case-sensitivity </title> </head> <body> <p id = "output"> <script> let char1 = 'S'; let char2 = 's' let ans = char1 == char2; document.getElementById("output").innerHTML += "s == S " + ans; </script> </body> </html>Output
s == S false
JavaScript Strings are Immutable
In JavaScript, you can't change the characters of the string. However, you can update the whole string.
Example
In the example below, we try to update the first character of the string, but it doesn't get updated, which you can see in the output.
After that, we update the whole string, and you can observe the changes in the string.
<html> <head> <title> JavaScript − Immutable String </title> </head> <body> <p id = "output"> </p> <script> const output = document.getElementById("output"); let str = "Animal"; str[0] = 'j'; output.innerHTML += "The string is: " + str + "<br>"; str = "Hi!"; output.innerHTML += "The updated string is: " + str; </script> </body> </html>Output
The string is: Animal The updated string is: Hi!
Escape Characters
You can use the special characters with the string using the backslash (\) characters. Here is the list of special characters.
Escape character | Description |
---|---|
\" | Double quote |
\' | Single quote |
\\ | Backslash |
\n | New line |
\t | Tab |
\b | backspace |
\f | Form feed |
\v | Verticle tab |
\r | Carriage return |
\uXXXX | Unicode escape |
Example
In the example below, we added a single quote between the characters of the str1 string and a backslash between the characters of the str2 string.
<html> <head> <title> JavaScript - Escape Characters </title> </head> <body> <p id = "output"> </p> <script> const output = document.getElementById("output"); let str1 = "Your\'s welcome!"; let str2 = "Backslash \\"; output.innerHTML += "str1 == " + str1 + "<br>"; output.innerHTML += "str2 == " + str2 + "<br>"; </script> </body> </html>Output
str1 == Your's welcome! str2 == Backslash \
String HTML Wrappers
Here is a list of the methods that return a copy of the string wrapped inside an appropriate HTML tag.
Sr.No. | Method & Description |
---|---|
1 | anchor()
Creates an HTML anchor that is used as a hypertext target. |
2 | big()
Creates a string to be displayed in a big font as if it were in a <big> tag. |
3 | blink()
Creates a string to blink as if it were in a <blink> tag. |
4 | bold()
Creates a string to be displayed as bold as if it were in a <b> tag. |
5 | fixed()
Causes a string to be displayed in fixed-pitch font as if it were in a <tt> tag |
6 | fontcolor()
Causes a string to be displayed in the specified color as if it were in a <font color="color"> tag. |
7 | fontsize()
Causes a string to be displayed in the specified font size as if it were in a <font size="size"> tag. |
8 | italics()
Causes a string to be italic, as if it were in an <i> tag. |
9 | link()
Creates an HTML hypertext link that requests another URL. |
10 | small()
Causes a string to be displayed in a small font, as if it were in a <small> tag. |
11 | strike()
Causes a string to be displayed as struck-out text, as if it were in a <strike> tag. |
12 | sub()
Causes a string to be displayed as a subscript, as if it were in a <sub> tag |
13 | sup()
Causes a string to be displayed as a superscript, as if it were in a <sup> tag |