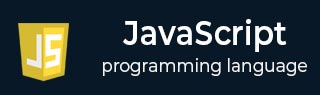
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Placement in HTML File
JavaScript Placement in HTML File
There is flexibility to place JavaScript code anywhere in an HTML document. However, the most preferred ways to include JavaScript in an HTML file are as follows −
Script in <head>...</head> section.
Script in <body>...</body> section.
Script in <body>...</body> and <head>...</head> sections.
Script in an external file and then include in <head>...</head> section.
You can follow the syntax below to add JavaScript code using the script tag.
<script> // JavaScript code </script>
In the following section, we will see how we can place JavaScript in an HTML file in different ways.
JavaScript in <head>...</head> section
If you want to have a script run on some event, such as when a user clicks somewhere,then you will place that script in the head as follows −
<html> <head> <script type = "text/javascript"> function sayHello() { alert("Hello World") } </script> </head> <body> <input type = "button" onclick = "sayHello()" value = "Say Hello" /> </body> </html>
JavaScript in <body>...</body> section
If you need a script to run as the page loads so that the script generates content in the page, then the script goes in the <body> portion of the document. In this case, youwould not have any function defined using JavaScript. Take a look at the following code.
<html> <head> </head> <body> <script type = "text/javascript"> document.write("Hello World") </script> <p>This is web page body </p> </body> </html>
JavaScript in <body> and <head> Sections
You can put your JavaScript code in <head> and <body> sections altogether as follows −
<html> <head> <script type = "text/javascript"> function sayHello() { alert("Hello World") } </script> </head> <body> <script type = "text/javascript"> document.write("Hello World") </script> <input type = "button" onclick = "sayHello()" value = "Say Hello" /> </body> </html>
JavaScript in External File
As you begin to work more extensively with JavaScript, you will likely find cases where you are reusing identical JavaScript code on multiple pages of a site.
You are not restricted to be maintaining identical code in multiple HTML files. The script tag provides a mechanism to allow you to store JavaScript in an external file and then include it in your HTML files.
To use JavaScript from an external file source, you need to write all your JavaScript source code in a simple text file with the extension ".js" and then include that file as shown below.
For example, you can keep the following content in the filename.js file, and then you can use the sayHello function in your HTML file after including the filename.js file.
filename.js
function sayHello() { alert("Hello World") }
External JavaScript file doesn’t contain the <script> tag.
Here is an example to show how you can include an external JavaScript file in your HTML code using the script tag and its src attribute.
You may include the external script reference within the <head> or <body> tag.
<html> <head> <script type = "text/javascript" src = "filename.js" ></script> </head> <body> ... </body> </html>
Also, you can create different modules to maintain code better and import each module in another JavaScript file or import all modules in a single HTML file.
You can follow the below code to add multiple scripts into a single HTML file.
<head> <script src = "filename1.js" ></script> <script src = "filename2.js" ></script> <script src = "filename3.js" ></script> </head>
External References
You can add an external JavaScript file in the HTML using the below 3 ways.
1. Using the full file path
When you need to add any hosted JavaScript file or a file that doesn’t exists in the same project into the HTML, you should use the full file path.
For example,
<head> <script src = "C://javascript/filename.js" ></script> </head>
2. Using the relative file path
If you are working on the project and JavaScript and HTML both files are in different folders, you can use the relative file path.
<head> <script src = "javascript\filename.js" ></script> </head>
3. Using the filename only
If HTML and JavaScript both files are in the same folder, you can use the file name.
<head> <script src = "filename.js" ></script> </head>
Advantages of using the <script> tag
Here are the advantages of using the <script> tag to add JavaScript in the HTML.
Ease of Integration
The <script> tag allows developers to integrate JavaScript into the HTML file easily. Adding JavaScript to the HTML file allows you to add behavior and interactivity to the web page.
Immediate Execution
Whenever the browser finds a <script> tag on the web page, it immediately executes the JavaScript code defined inside that. It enables website visitors to interact with the web pages and get real-time updates immediately.
Inline and External scripts
You can use the <script> tag to add the inline or external script into the HTML file. If you want to load JavaScript before the HTML of a web page, you can add the <script. Tag in the <head> tag. Otherwise, you can add the <script> tag in the <body> tag.
External Libraries and Frameworks integration
The <script> tag enables you to add external libraries and frameworks to the HTML web page.
For example, in the below code, we have added JQuery to the web page using its CDN.
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.7.0/jquery.min.js"> </script>
Here, the "src" attribute contains the hosted link to the library.
Global Scope Access
Whatever code you define in the script tag has access to the global scope of the web page. You can access the global variables, functions, etc., anywhere in the code.