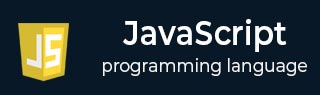
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Function apply() Method
Function apply() Method
The Function apply() method in JavaScript allows us to invoke a function given a specific value for this and arguments provided as an array.
The Function call() and apply() methods are very similar, but the main difference between them is function apply() method takes a single array containing all function arguments, and the function call() method takes individual arguments.
Same as the Function call() method, we can use the apply() method to manipulate the this value and can assign an orbitrary object to this.
Syntax
The syntax of the Function apply() method in JavaScriot is as follows −
func.apply(thisArg, [arg1, arg2, ... argN]);
Parameters
thisArg − The 'thisArg' grument represents the function context. It is an object whose properties are needed to access the reference function using the 'this' keyword.
[arg1, arg2, ... argN] − They are arguments to pass to the function.
Return value
It returns the resultant value returned from the function.
Examples
Let's understand JavaScript Function apply() method with the help of some examples.
Using apply() method without passing argument
In the below code, we have defined the test() function printing the message in the output.
We invoked the function in a standard way and used the apply() method without passing any argument to invoke the function. The output shows that the apply() method gives the same output as a normal function call.
<html> <head> <title> JavaScript - Function apply() method </title> </head> <body> <p id = "output1"> </p> <p id = "output2"> </p> <script> function test() { return "The function test is invoked!"; } document.getElementById("output1").innerHTML = test(); document.getElementById("output2").innerHTML = test.apply(); </script> </body> </html>
Output
The function test is invoked! The function test is invoked!
Using apply() method with this argument only
In the below code, the 'car' object contains three different properties. We passed the car object as a 'thisArg' argument of the apply() method.
In the showCar() function, we access the properties of the car object using the 'this' keyword and print it into the output.
<html> <head> <title> JavaScript - Function apply() method </title> </head> <body> <p id = "output"> </p> <script> function showCar() { return "The price of the " + this.name + " " + this.model + " is: " + this.price; } let car = { name: "OD", model: "Q6", price: 10000000, } document.getElementById("output").innerHTML = showCar.apply(car); </script> </body> </html>
Output
The price of the OD Q6 is: 10000000
Using apply() method with an array of function arguments
In the example below, we pass the nums object as the first argument of the apply() method. After that, we pass the array of arguments as an apply() method's argument.
In the printSum() function, we access the object properties using the 'this' keyword and function arguments using the function parameters.
<html> <head> <title> JavaScript - Function apply() method </title> </head> <body> <p id = "output"> </p> <script> function printSum(p1, p2) { return this.num1 + this.num2 + p1 + p2; } const nums = { num1: 5, num2: 10, } const ans = printSum.apply(nums, [40, 32]); document.getElementById("output").innerHTML = "Total sum is " + ans; </script> </body> </html>
Output
Total sum is 87
Using apply() method with built-in functions
You can also use the apply() method with built-in object methods. We can invoke the built-in object methods (such as Math.max or Math.min) using apply() method.
In the example below, we use apply() method with built-in JavaScript functions - Math.max and Math.min. We can't directly use these methods for the arrays. We invoke the Math.max and Math.min methods using apply() method. We pass null as thisArg and the array of five integers as the function argument.
<html> <head> <title> JavaScript - Function apply() method </title> </head> <body> <p id = "output-max"> Max element in the array: </p> <p id = "output-min"> Max element in the array:</p> <script> const numbers = [7, 6, 4, 3, 9]; document.getElementById("output-max").innerHTML += Math.max.apply(null, numbers); document.getElementById("output-min").innerHTML += Math.min.apply(null, numbers); </script> </body> </html>
Output
Max element in the array: 9 Max element in the array:3
Notice if you use Math.max() or Math.min() methods directly for arrays to find max or min element in the array, the result will be NaN.