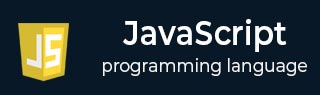
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Function bind() Method
Function bind() Method
The function bind() method in JavaScript creates a new function with a specified this value and optional arguments, without invoking the original function immediately. It is commonly used to set the context (this) for a function and partially apply arguments. This method is used to bind a particular object to a common function.
To understand the function bind() method, we should first understand the "this" keyword. In JavaScript (and other programming languages also), this is a special keyword that is used within functions to refer to the object on which the function is invoked. The value of this is depended on how a function is being called and behaviour of this can vary in different contexts.
Syntax
The syntax of JavaScript function bind() method is as follows −
functionToBind.bind(thisValue, arg1, arg2, ...);
Here functionToBind is the original function whose this value and arguments you want to set.
Parameters
thisValue − The value that should be passed as the this parameter when the new function is called.
arg1, arg2, ... − Optional arguments that will be fixed (partially applied) when the new function is called. These arguments will be prepended to the arguments provided to the new function.
Lets now understand the Function bind() method with the help of some program examples.
Without Function bind() Method
Here we will create a general and common function greet() which simply prints to the console. We create a constant object named person and give it some property i.e. name and we then invoke the function greet by passing it a message "Hello".
<html> <body> <div id = "demo"> </div> <script> const output = document.getElementById("demo"); function greet(message) { output.innerHTML = message + ', ' + this.name; } const person = { name: 'John Doe' }; greet('Hello'); </script> </body> </html>
Output
Hello,
In this example, when the greet function is called directly without using bind, the this value is not explicitly set, leading to an undefined or global object (window in a browser environment) being used as this.
With Function bind() method
To overcome the issue in the previous code where it could not fetch any associated name, we make use of the bind function to bind the object person to the function greet.
<html> <body> <div id = "demo"> </div> <script> const output = document.getElementById("demo"); // Original function function greet(message) { output.innerHTML = message + ', ' + this.name; } // Object with a 'name' property const person = { name: 'John Doe' }; const greetPerson = greet.bind(person, 'Hello'); greetPerson(); </script> </body> </html>
Output
Hello, John Doe
The bind method was able to create a new function greetPerson wherein the this value has been explicitly set to the person object and argument "Hello" is partially applied as in the previous code as well.
Using bind() ensures that the function is executed in the desired context, preventing issues related to the default behaviour of this in JavaScript functions.
Example: Binding different objects to same function
We have created three objects with x and y coordinates of a point, a function printVal is created to print to the console the coordinates of the point. The bind method binds the points to the printVal function and prints the x, y coordinates of each of the points.
<html> <body> <div id = "demo"> </div> <script> const output = document.getElementById("demo"); const points1 = { X: 100, Y: 100 } const points2 = { X: 200, Y: 100 } const points3 = { X: 350, Y: 400 } function printVal() { output.innerHTML += "Coordinates: "+this.X + "," + this.Y + "<br>"; } const printFunc2 = printVal.bind(points1); printFunc2(); const printFunc3 = printVal.bind(points2); printFunc3(); const printFunc4 = printVal.bind(points3); printFunc4(); </script> </body> </html>
Output
Coordinates: 100,100 Coordinates: 200,100 Coordinates: 350,400
Example: Setting the default values of function parameters
This is a new scenario wherein we make use of the bind function to predefine the parameter. The multiply() function simply takes 2 inputs and returns their product. By using the bind() method we can set any of the parameters to a default value as needed.
In the below example, it sets the variable y to 2 and hence upon calling the function by passing just 1 parameter i.e. x as 5 it gets multiplies by the preset 2 and returns the output of 5x2=10.
<html> <body> <div id = "output"> </div> <script> // Original function with parameters function multiply(x, y) { return x * y; } // Using bind to preset the first parameter const multiplyByTwo = multiply.bind(null, 2); // Calling the bound function with only the second parameter document.getElementById("output").innerHTML= multiplyByTwo(5); </script> </body> </html>
Output
10
It is important to note that the bind method creates and returns a new function and does not modify the original function. The same function can be reused and yet made to match different use cases without actually modifying.