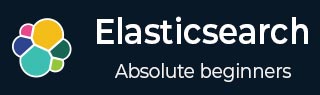
- Elasticsearch Tutorial
- Elasticsearch - Home
- Elasticsearch - Basic Concepts
- Elasticsearch - Installation
- Elasticsearch - Populate
- Migration between Versions
- Elasticsearch - API Conventions
- Elasticsearch - Document APIs
- Elasticsearch - Search APIs
- Elasticsearch - Aggregations
- Elasticsearch - Index APIs
- Elasticsearch - CAT APIs
- Elasticsearch - Cluster APIs
- Elasticsearch - Query DSL
- Elasticsearch - Mapping
- Elasticsearch - Analysis
- Elasticsearch - Modules
- Elasticsearch - Index Modules
- Elasticsearch - Ingest Node
- Elasticsearch - Managing Index Lifecycle
- Elasticsearch - SQL Access
- Elasticsearch - Monitoring
- Elasticsearch - Rollup Data
- Elasticsearch - Frozen Indices
- Elasticsearch - Testing
- Elasticsearch - Kibana Dashboard
- Elasticsearch - Filtering by Field
- Elasticsearch - Data Tables
- Elasticsearch - Region Maps
- Elasticsearch - Pie Charts
- Elasticsearch - Area and Bar Charts
- Elasticsearch - Time Series
- Elasticsearch - Tag Clouds
- Elasticsearch - Heat Maps
- Elasticsearch - Canvas
- Elasticsearch - Logs UI
- Elasticsearch Useful Resources
- Elasticsearch - Quick Guide
- Elasticsearch - Useful Resources
- Elasticsearch - Discussion
Elasticsearch - API Conventions
Application Programming Interface (API) in web is a group of function calls or other programming instructions to access the software component in that particular web application. For example, Facebook API helps a developer to create applications by accessing data or other functionalities from Facebook; it can be date of birth or status update.
Elasticsearch provides a REST API, which is accessed by JSON over HTTP. Elasticsearch uses some conventions which we shall discuss now.
Multiple Indices
Most of the operations, mainly searching and other operations, in APIs are for one or more than one indices. This helps the user to search in multiple places or all the available data by just executing a query once. Many different notations are used to perform operations in multiple indices. We will discuss a few of them here in this chapter.
Comma Separated Notation
POST /index1,index2,index3/_search
Request Body
{ "query":{ "query_string":{ "query":"any_string" } } }
Response
JSON objects from index1, index2, index3 having any_string in it.
_all Keyword for All Indices
POST /_all/_search
Request Body
{ "query":{ "query_string":{ "query":"any_string" } } }
Response
JSON objects from all indices and having any_string in it.
Wildcards ( * , + , –)
POST /school*/_search
Request Body
{ "query":{ "query_string":{ "query":"CBSE" } } }
Response
JSON objects from all indices which start with school having CBSE in it.
Alternatively, you can use the following code as well −
POST /school*,-schools_gov /_search
Request Body
{ "query":{ "query_string":{ "query":"CBSE" } } }
Response
JSON objects from all indices which start with “school” but not from schools_gov and having CBSE in it.
There are also some URL query string parameters −
- ignore_unavailable − No error will occur or no operation will be stopped, if the one or more index(es) present in the URL does not exist. For example, schools index exists, but book_shops does not exist.
POST /school*,book_shops/_search
Request Body
{ "query":{ "query_string":{ "query":"CBSE" } } }
Request Body
{ "error":{ "root_cause":[{ "type":"index_not_found_exception", "reason":"no such index", "resource.type":"index_or_alias", "resource.id":"book_shops", "index":"book_shops" }], "type":"index_not_found_exception", "reason":"no such index", "resource.type":"index_or_alias", "resource.id":"book_shops", "index":"book_shops" },"status":404 }
Consider the following code −
POST /school*,book_shops/_search?ignore_unavailable = true
Request Body
{ "query":{ "query_string":{ "query":"CBSE" } } }
Response (no error)
JSON objects from all indices which start with school having CBSE in it.
allow_no_indices
true value of this parameter will prevent error, if a URL with wildcard results in no indices. For example, there is no index that starts with schools_pri −
POST /schools_pri*/_search?allow_no_indices = true
Request Body
{ "query":{ "match_all":{} } }
Response (No errors)
{ "took":1,"timed_out": false, "_shards":{"total":0, "successful":0, "failed":0}, "hits":{"total":0, "max_score":0.0, "hits":[]} }
expand_wildcards
This parameter decides whether the wildcards need to be expanded to open indices or closed indices or perform both. The value of this parameter can be open and closed or none and all.
For example, close index schools −
POST /schools/_close
Response
{"acknowledged":true}
Consider the following code −
POST /school*/_search?expand_wildcards = closed
Request Body
{ "query":{ "match_all":{} } }
Response
{ "error":{ "root_cause":[{ "type":"index_closed_exception", "reason":"closed", "index":"schools" }], "type":"index_closed_exception", "reason":"closed", "index":"schools" }, "status":403 }
Date Math Support in Index Names
Elasticsearch offers a functionality to search indices according to date and time. We need to specify date and time in a specific format. For example, accountdetail-2015.12.30, index will store the bank account details of 30th December 2015. Mathematical operations can be performed to get details for a particular date or a range of date and time.
Format for date math index name −
<static_name{date_math_expr{date_format|time_zone}}> /<accountdetail-{now-2d{YYYY.MM.dd|utc}}>/_search
static_name is a part of expression which remains the same in every date math index like account detail. date_math_expr contains the mathematical expression that determines the date and time dynamically like now-2d. date_format contains the format in which the date is written in index like YYYY.MM.dd. If today’s date is 30th December 2015, then <accountdetail-{now-2d{YYYY.MM.dd}}> will return accountdetail-2015.12.28.
Expression | Resolves to |
---|---|
<accountdetail-{now-d}> | accountdetail-2015.12.29 |
<accountdetail-{now-M}> | accountdetail-2015.11.30 |
<accountdetail-{now{YYYY.MM}}> | accountdetail-2015.12 |
We will now see some of the common options available in Elasticsearch that can be used to get the response in a specified format.
Pretty Results
We can get response in a well-formatted JSON object by just appending a URL query parameter, i.e., pretty = true.
POST /schools/_search?pretty = true
Request Body
{ "query":{ "match_all":{} } }
Response
…………………….. { "_index" : "schools", "_type" : "school", "_id" : "1", "_score" : 1.0, "_source":{ "name":"Central School", "description":"CBSE Affiliation", "street":"Nagan", "city":"paprola", "state":"HP", "zip":"176115", "location": [31.8955385, 76.8380405], "fees":2000, "tags":["Senior Secondary", "beautiful campus"], "rating":"3.5" } } ………………….
Human Readable Output
This option can change the statistical responses either into human readable form (If human = true) or computer readable form (if human = false). For example, if human = true then distance_kilometer = 20KM and if human = false then distance_meter = 20000, when response needs to be used by another computer program.
Response Filtering
We can filter the response to less fields by adding them in the field_path parameter. For example,
POST /schools/_search?filter_path = hits.total
Request Body
{ "query":{ "match_all":{} } }
Response
{"hits":{"total":3}}