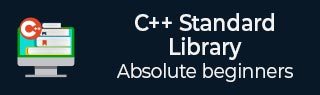
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Utility Library - swap Function
Description
It exchanges the values of a and b.
Declaration
Following is the declaration for std::swap function.
template <class T> void swap (T& a, T& b);
C++11
template <class T> void swap (T& a, T& b) noexcept (is_nothrow_move_constructible<T>::value && is_nothrow_move_assignable<T>::value);
Parameters
a, b − These are two objects.
Return Value
none
Exceptions
Basic guarantee − if the construction or assignment of type T throws.
Data races
Both a and b are modified.
Example
In below example explains about std::swap function.
#include <iostream> #include <utility> int main () { int foo[4]; int bar[] = {100,200,300,400}; std::swap(foo,bar); std::cout << "foo contains:"; for (int i: foo) std::cout << ' ' << i; std::cout << '\n'; return 0; }
Let us compile and run the above program, this will produce the following result −
foo contains: 100 200 300 400
utility.htm
Advertisements
To Continue Learning Please Login