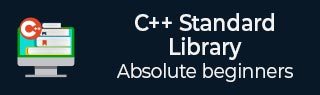
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Set Library - get_allocator Function
Description
It returns a copy of the allocator object associated with the set.
Declaration
Following are the ways in which std::set::get_allocator works in various C++ versions.
C++98
allocator_type get_allocator() const;
C++11
allocator_type get_allocator() const noexcept;
Return value
It returns a copy of the allocator object associated with the set.
Exceptions
If an exception is thrown, there are no changes in the container.
Time complexity
Time complexity depens on logarithmic.
Example
The following example shows the usage of std::set::get_allocator.
#include <iostream> #include <set> int main () { std::set<int> myset; int * p; unsigned int i; p = myset.get_allocator().allocate(5); for (i = 0; i < 5; i++) p[i]=(i+1)*10; std::cout << "The allocated array contains:"; for (i = 0; i < 5; i++) std::cout << ' ' << p[i]; std::cout << '\n'; myset.get_allocator().deallocate(p,5); return 0; }
The above program will compile and execute properly.
The allocated array contains: 10 20 30 40 50
set.htm
Advertisements