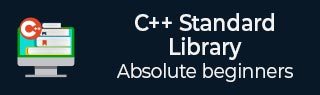
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Functional Library - constructor
Description
It constructs a std::function from a variety of sources.
Declaration
Following is the declaration for std::function.
C++11
The following are creating an empty function.
function(); function( std::nullptr_t );
Exceptions
noexcept:noexcept specification.
Copy and Move
The following functions are Copying or moving the target of other to the target of *this. If other is empty, *this will be empty after the call too.
function( const function& other ); function( function&& other );
Exceptions
noexcept:noexcept specification.
Initialize the target
The following functions is initializing the target with a copy of f. If f is a null pointer to function or null pointer to member, *this will be empty after the call. This constructor does not participate in overload resolution unless f is Callable for argument types Args and return type R
template< class F > function( F f );
Exceptions
noexcept:noexcept specification.
Allowcate the memory
The following functions are same as just that alloc is used to allocate memory for any internal data structures that the function might use.
template< class Alloc > function( std::allocator_arg_t, const Alloc& alloc ); template< class Alloc > function( std::allocator_arg_t, const Alloc& alloc, std::nullptr_t ); template< class Alloc > function( std::allocator_arg_t, const Alloc& alloc, const function& other ); template< class Alloc > function( std::allocator_arg_t, const Alloc& alloc, function&& other ); template< class F, class Alloc > function( std::allocator_arg_t, const Alloc& alloc, F f );
Exceptions
noexcept:noexcept specification.
Parameters
other − This function object used to initialize *this.
f − a callable used to initialize *this.
alloc − It is used for internal memory allocation.