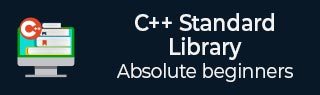
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Atomic Library - fetch add
Description
It automically atomically adds the argument to the value stored in the atomic object and obtains the value held previously.
Declaration
Following is the declaration for std::atomic::fetch_add.
T fetch_add (T val, memory_order sync = memory_order_seq_cst) volatile noexcept;
C++11
T fetch_add (T val, memory_order sync = memory_order_seq_cst) noexcept;
Following is the declaration for std::atomic::fetch_add(member only of atomic
T fetch_add (ptrdiff_t val, memory_order sync = memory_order_seq_cst) volatile noexcept;
C++11
T fetch_add (ptrdiff_t val, memory_order sync = memory_order_seq_cst) noexcept;
Parameters
arg − It is used put the other argument of arithmetic addition.
order − It is used enforce the memory order for the value.
Return Value
It returns the value immediately preceding the effects of this function in the modification order of *this.
Exceptions
No-noexcept − this member function never throws exceptions.
Example
In below example for std::atomic::fetch_add.
#include <iostream> #include <thread> #include <atomic> std::atomic<long long> data; void do_work() { data.fetch_add(1, std::memory_order_relaxed); } int main() { std::thread th1(do_work); std::thread th2(do_work); std::thread th3(do_work); std::thread th4(do_work); std::thread th5(do_work); th1.join(); th2.join(); th3.join(); th4.join(); th5.join(); std::cout << "Ans:" << data << '\n'; }
The sample output should be like this −
Ans:5
atomic.htm
Advertisements