
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 6704 Articles for Javascript

619 Views
JavaScript provides us with different methods to check whether a string contains a substring or not. In this article, we use the following three methods for this purpose-String search() methodString indexOf() methodString includes() methodLet’s explore in detail each method.1. String search() methodThe string search method can be used to search a text (using regular expression) in a string.It matches the string against a regular expression and returns the index (position) of the first match.It returns -1 if no match is found. It’s case-sensitive.The string search() method is an ES1 feature. It is fully supported in all browsers.Syntaxstring.search(searchValue)searchValue is a regular ... Read More

548 Views
The “use strict” is a directive, which is a literal expression. It was introduced in JavaScript 1.8.5. As the name suggests, “use strict” indicates that the code is to be executed in strict mode.Benefits of using “use strict”It’s easier to write "secure" JavaScript codes.It changes previously accepted "bad syntax" into real errors. As an example, mistyping a variable name creates a new global variable. When using strict mode, this will throw an error. It leads to making it impossible to accidentally create a global variable.A programmer will not receive any error feedback assigning values to non-writable properties.But in strict mode, ... Read More

16K+ Views
The window.location object contains the current location or URL information. We can redirect the users to another webpage using the properties of this object. window.location can be written without the prefix window.We use the following properties of the window.location object to redirect the users to another webpage −window.location.href- it returns the URL (href) of the current page.window.location.replace()- it replaces the current document with new document.window.location.assign() loads a new document.The syntaxes below are used to redirect to another web page. We omit the window prefix and use only location in all the program examples. You can try running the programs using ... Read More

77 Views
Double equals (==) is abstract equality comparison operator, which transforms the operands to the same type before making the comparison.For example,4 == 4 // true '4' == 4 // true 4 == '4' // true 0 == false // trueTriple equals (===) are strict equality comparison operator, which returns false for different types and different content.For example,4 === 4 // true 4 === '4' // false var v1 = {'value': 'key'}; var v2 = {'value': 'key'}; v1 === v2 //false

100 Views
To remove a property from a JavaScript object, use the delete keyword. You can try to run the following code to learn how to remove a propertyExampleLive Demo var cricketer = { name:"Amit", rank:1, points: 150 }; delete cricketer.rank; document.getElementById("demo").innerHTML = cricketer.name + " has " + cricketer.rank + " rank.";

408 Views
The window.location object can be used to get the current URL.window.location.href returns the href(URL) of the current page.I am using these code in my MVC projet.Example var iid=document.getElementById("dd"); alert(window.location.href); iid.innerHTML = "URL is" + window.location.href;

11K+ Views
Suppose you are building a web app that manages the data of daily tasks, the user inserts the task and a list of tasks gets formed. But at some point in time, the user wants to remove all the tasks from the list and wants to make the list empty. So, as a developer, you should know how to remove all the child elements from the DOM node. To remove all the elements from the DOM node we have the following approaches. By iterating the DOM nodes and using the removeChild method. By Erasing the innerHTML value to a ... Read More
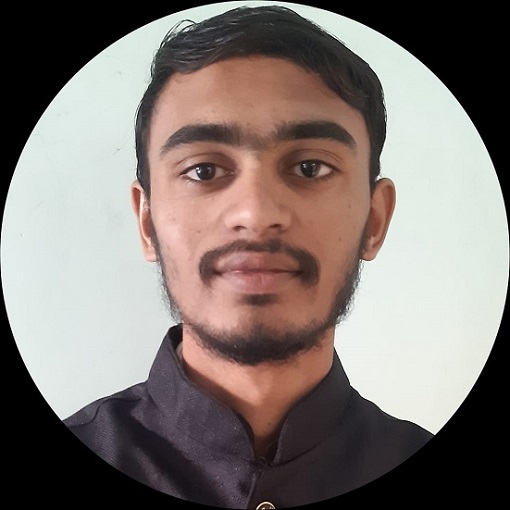
1K+ Views
Let us first understand what is JavaScript objects and JavaScript events. An object in JavaScript is a distinct entity having properties and a type. For an instance, contrast it with a bowl. A bowl is an object with some properties. The properties are design, color, material, weight, etc. Like this, JavaScript object also has some properties. An independent entity known as a JavaScript object can store numerous values as its properties and methods. While a method represents a function, an object property maintains a literal value. Either the object literal or the object function object() { [native code] } syntax ... Read More

2K+ Views
Use event delegation to include buttons for both add a new and delete a table row on a web page using jQuery.Firstly, set the delete button:XTo fire event on click of a button, use jQuery on() method:$(document).on('click', 'button.deletebtn', function () { $(this).closest('tr').remove(); return false; });The following is the complete code delete a row from a table using jQuery:Example Live Demo $(document).ready(function(){ var x = 1; $("#newbtn").click(function () { $("table tr:first").clone().find("input").each(function () { $(this).val('').attr({ ... Read More

2K+ Views
To add a new list element under a ul element, use the jQuery append() methodSet input type text initiallyValue: Now on the click of a button, add a new list element using the val() and append() methods in jQuery:$('button').click(function() { var mylist = $('#input').val(); $('#list').append(''+mylist+''); return false; });You can try to run the following code to learn how to add a new list element using jQuery:Example Live Demo $(document).ready(function(){ $('button').click(function() { var mylist = $('#input').val(); ... Read More
To Continue Learning Please Login