
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 10877 Articles for Web Development

109 Views
Definitely, the following way of declaring multiple variables is more efficient:var variable1 = 5; var variable2 = 3.6; var variable3 = “Amit”;Suppose you need to add, remove, or update a variable, then you can easily do it.But with the following method, you need to do more changes. For example, on removing a variable, you need to remove the semi-colon. If it’s the first, then you need to add var to the second variable.var variable1 = 5, variable2 = 3.6, variable3 = “Amit”
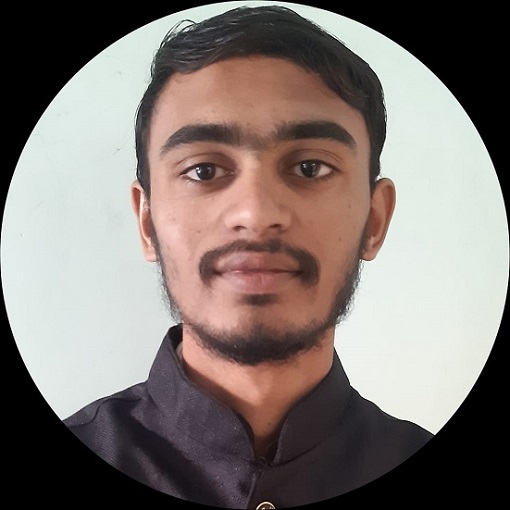
949 Views
In this tutorial, we will look at a few ways to prevent duplicate JavaScript variable declarations and comparison between them from understanding which one is suitable in a given context. The best way to prevent duplicate variable declaration is to avoid creating global variables. Let’s move forward to discuss this. Wrapping Code Within a Function Here, the variables declared inside the function are not accessible outside the function and vice versa. Users can follow the syntax below for using this method. Syntax (function(){ var varNam = "test"; }()) Here varNam is wrapped inside a function. Example ... Read More
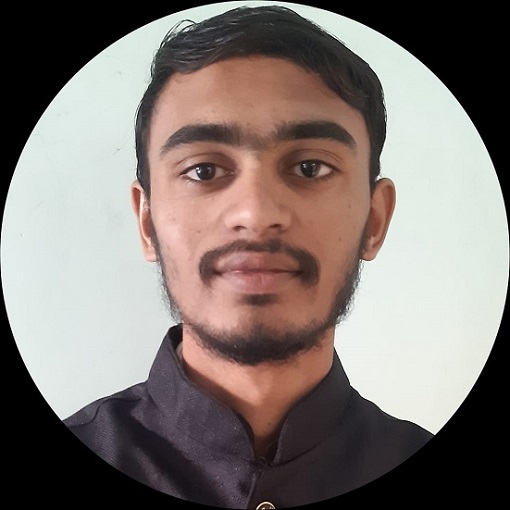
3K+ Views
In this tutorial, we will learn different approaches to checking if a JavaScript variable is of function type or not. In JavaScript, the function contains the block of code, making the code reusability better.There are mainly two ways to declare the functions, one is a named function, and another is an anonymous function. While declaring the anonymous function, we need to store it in some variable to access it from somewhere in our code or call that function.Here, users can see how to declare the anonymous function and store it into the variable.let tutorialspoint = function() { //code for the ... Read More

15K+ Views
To unset a variable in JavaScript, use the undefined. After that, use delete operator to completely remove it.Let’s try the code snippet again.var str = “Demo Text”; window.onscroll = function() { var y = this.pageYOffset; if(y > 200) { document.write(nxt); nxt = undefined; // unset delete(nxt); // this removes the variable completely document.write(nxt); // the result will be undefined } }

546 Views
On getting the result of the following, you can find whether a variable is null or undefined. If the result is “false”, it means the variable is null and undefined.Here, the variable results in “True” − var age = 10; if(age) { document.write("True"); } else { document.write("False"); }
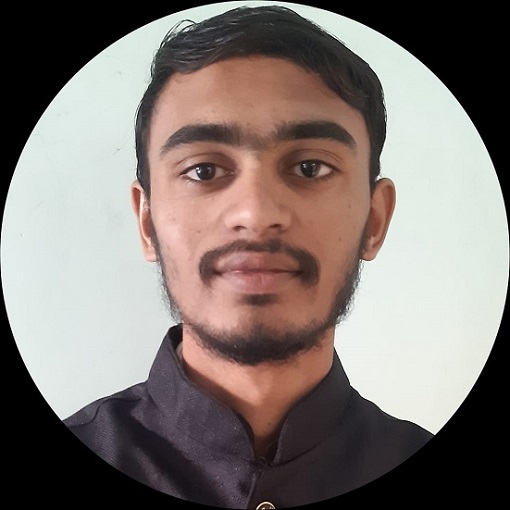
5K+ Views
In this article, we will learn what characters are valid for JavaScript variable names. We use variables to save values. First, we have to define the variable, and then we can assign a value to it. We can use the variable name to access the variable in the code. With a variable name, we can reassign its value. As you adhere to a few principles, variable names are quite versatile. Rules A letter, dollar sign($), or underscore (_) must make up the first character. A number cannot be the initial character. Any letter, number, or underscore can complete the ... Read More
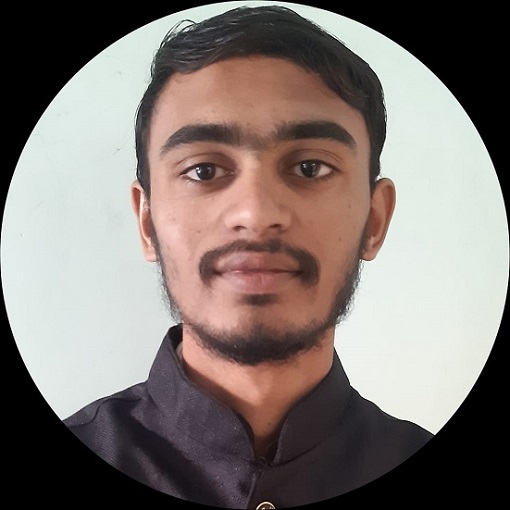
14K+ Views
In this tutorial, we will learn to check for null, undefined, or blank variables in JavaScript. There is no particular built-in library function to check whether a variable is undefined or null. Still, we can use some operators such as typeof operator and equality operator to check the variable. Before we proceed with the tutorial, let’s clear the doubt between the null, undefined, or blank variable. Null VS Undefined We can say that the Null and undefined variables are almost the same but not identical. Undefined variable − It is a variable not declared in the program, and the ... Read More

115 Views
To check if a variable is an array, use “instanceof. The following is the syntax −variable instanceof ArrayExampleLet’s seen an example to check if the variable “sports” is an array or not? var sports = [ "tennis", "football", "cricket" ]; if (sports instanceof Array) { alert('Array!'); } else { alert('Not an array'); }

254 Views
When you will begin working about jQuery, you will get to know about the usage of the $ sign. A $ sign is used to define jQuery.jQuery variables begin with $ to distinguish them from a standard JavaScript object. Also, it is a convention. jQuery selectors start with the dollar sign and parentheses() − $.Let's take an Example −// jQuery object var $phone = $("#myphone"); // dom object var phone_el = $("#myphone").get(1);

213 Views
The delete operator in JavaScript is used to remove properties from an object to remove element(s) from an array or to remove element(s) from a set object. Always remember the following points about delete: delete is a keyword in JavaScript, so it shouldn’t be altered, and you cannot use delete objects in single variables since it is used only for deleting properties of objects. Let's look in detail at how we can implement delete operators in JavaScript. Deleting object properties Objects in JavaScript, we will write using key-value pair like const Employee = { firstname: 'Devika', ... Read More
To Continue Learning Please Login