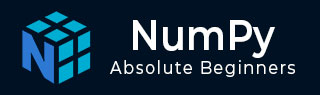
- NumPy Tutorial
- NumPy - Home
- NumPy - Introduction
- NumPy - Environment
- NumPy - Ndarray Object
- NumPy - Data Types
- NumPy - Array Attributes
- NumPy - Array Creation Routines
- NumPy - Array from Existing Data
- Array From Numerical Ranges
- NumPy - Indexing & Slicing
- NumPy - Advanced Indexing
- NumPy - Broadcasting
- NumPy - Iterating Over Array
- NumPy - Array Manipulation
- NumPy - Binary Operators
- NumPy - String Functions
- NumPy - Mathematical Functions
- NumPy - Arithmetic Operations
- NumPy - Statistical Functions
- Sort, Search & Counting Functions
- NumPy - Byte Swapping
- NumPy - Copies & Views
- NumPy - Matrix Library
- NumPy - Linear Algebra
- NumPy - Matplotlib
- NumPy - Histogram Using Matplotlib
- NumPy - I/O with NumPy
- NumPy Useful Resources
- NumPy Compiler
- NumPy - Quick Guide
- NumPy - Useful Resources
- NumPy - Discussion
numpy.insert
This function inserts values in the input array along the given axis and before the given index. If the type of values is converted to be inserted, it is different from the input array. Insertion is not done in place and the function returns a new array. Also, if the axis is not mentioned, the input array is flattened.
The insert() function takes the following parameters −
numpy.insert(arr, obj, values, axis)
Where,
Sr.No. | Parameter & Description |
---|---|
1 | arr Input array |
2 | obj The index before which insertion is to be made |
3 | values The array of values to be inserted |
4 | axis The axis along which to insert. If not given, the input array is flattened |
Example
import numpy as np a = np.array([[1,2],[3,4],[5,6]]) print 'First array:' print a print '\n' print 'Axis parameter not passed. The input array is flattened before insertion.' print np.insert(a,3,[11,12]) print '\n' print 'Axis parameter passed. The values array is broadcast to match input array.' print 'Broadcast along axis 0:' print np.insert(a,1,[11],axis = 0) print '\n' print 'Broadcast along axis 1:' print np.insert(a,1,11,axis = 1)
Its output would be as follows −
First array: [[1 2] [3 4] [5 6]] Axis parameter not passed. The input array is flattened before insertion. [ 1 2 3 11 12 4 5 6] Axis parameter passed. The values array is broadcast to match input array. Broadcast along axis 0: [[ 1 2] [11 11] [ 3 4] [ 5 6]] Broadcast along axis 1: [[ 1 11 2] [ 3 11 4] [ 5 11 6]]
numpy_array_manipulation.htm
Advertisements