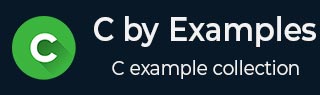
- Learn C By Examples Time
- Learn C by Examples - Home
- C Examples - Simple Programs
- C Examples - Loops/Iterations
- C Examples - Patterns
- C Examples - Arrays
- C Examples - Strings
- C Examples - Mathematics
- C Examples - Linked List
- C Programming Useful Resources
- Learn C By Examples - Quick Guide
- Learn C By Examples - Resources
- Learn C By Examples - Discussion
Program To Find LCM In C
L.C.M. or Least Common Multiple of two values, is the smallest positive value which the multiple of both values.
For example multiples of 3 and 4 are −
3 → 3, 6, 9, 12, 15 ...
4 → 4, 8, 12, 16, 20 ...
The smallest multiple of both is 12, hence the LCM of 3 and 4 is 12.
Algorithm
Algorithm of this program can be derived as −
START Step 1 → Initialize A and B with positive integers Step 2 → Store maximum of A & B to max Step 3 → Check if max is divisible by A and B Step 4 → If divisible, Display max as LCM Step 5 → If not divisible then step increase max, goto step 3 STOP
Pseudocode
Let's now derive pseudocode for this program −
procedure even_odd() Initialize A and B max = max(A, B) WHILE TRUE IF max is divisible by A and B THEN LCM = max BREAK ENDIF Increment max END WHILE DISPLAY LCM end procedure
Implementation
Implementation of this algorithm is given below −
#include<stdio.h> int main() { int a, b, max, step, lcm; a = 3; b = 4; lcm = 0; if(a > b) max = step = a; else max = step = b; while(1) { if(max%a == 0 && max%b == 0) { lcm = max; break; } max += step; } printf("LCM is %d", lcm); return 0; }
Output
Output of the program should be −
LCM is 12
mathematical_programs_in_c.htm
Advertisements