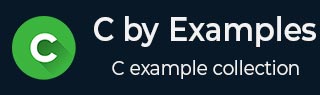
- Learn C By Examples Time
- Learn C by Examples - Home
- C Examples - Simple Programs
- C Examples - Loops/Iterations
- C Examples - Patterns
- C Examples - Arrays
- C Examples - Strings
- C Examples - Mathematics
- C Examples - Linked List
- C Programming Useful Resources
- Learn C By Examples - Quick Guide
- Learn C By Examples - Resources
- Learn C By Examples - Discussion
Program to find largest array element in C
Finding largest value in an array is a classic C array program. This program gives you an insight of iteration, array and conditional operators. We iteratively check each element of an array if it is the largest. See the below program.
Algorithm
Let's first see what should be the step-by-step procedure of this program −
START Step 1 → Take an array A and define its values Step 2 → Declare largest as integer Step 3 → Set 'largest' to 0 Step 4 → Loop for each value of A Step 5 → If A[n] > largest, Assign A[n] to largest Step 6 → After loop finishes, Display largest as largest element of array STOP
Pseudocode
Let's now see the pseudocode of this algorithm −
procedure largest_array(A) Declare largest as integer Set largest to 0 FOR EACH value in A DO IF A[n] is greater than largest THEN largest ← A[n] ENDIF END FOR Display largest end procedure
Implementation
This pseodocode can now be implemented in the C program as follows −
#include <stdio.h> int main() { int array[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 0}; int loop, largest; largest = array[0]; for(loop = 1; loop < 10; loop++) { if( largest < array[loop] ) largest = array[loop]; } printf("Largest element of array is %d", largest); return 0; }
The output should look like this −
Largest element of array is 9
array_examples_in_c.htm
Advertisements