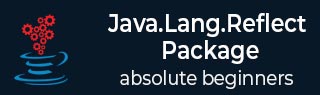
- java.lang.reflect Package Classes
- java.lang.reflect - Home
- java.lang.reflect - AccessibleObject
- java.lang.reflect - Array
- java.lang.reflect - Constructor<T>
- java.lang.reflect - Field
- java.lang.reflect - Method
- java.lang.reflect - Modifier
- java.lang.reflect - Proxy
- java.lang.reflect Package Extras
- java.lang.reflect - Interfaces
- java.lang.reflect - Exceptions
- java.lang.reflect - Error
- java.lang.reflect Useful Resources
- java.lang.reflect - Quick Guide
- java.lang.reflect - Useful Resources
- java.lang.reflect - Discussion
java.lang.reflect - Method Class
Introduction
The java.lang.reflect.Method class provides information about, and access to, a single method on a class or interface. The reflected method may be a class method or an instance method (including an abstract method). A Method permits widening conversions to occur when matching the actual parameters to invoke with the underlying method's formal parameters, but it throws an IllegalArgumentException if a narrowing conversion would occur.
Class declaration
Following is the declaration for java.lang.reflect.Method class −
public final class Method<T> extends AccessibleObject implements GenericDeclaration, Member
Class methods
Sr.No. | Method & Description |
---|---|
1 | boolean equals(Object obj)
Compares this Method against the specified object. |
2 | <T extends Annotation> T getAnnotation(Class<T> annotationClass)
Returns this element's annotation for the specified type if such an annotation is present, else null. |
3 | Annotation[] getDeclaredAnnotations()
Returns all annotations that are directly present on this element. |
4 | Class<T> getDeclaringClass()
Returns the Class object representing the class that declares the method represented by this Method object. |
5 | Object getDefaultValue()
Returns the default value for the annotation member represented by this Method instance. |
6 | Class<?>[] getExceptionTypes()
Returns an array of Class objects that represent the types of exceptions declared to be thrown by the underlying constructor represented by this Constructor object. |
7 | Type[] getGenericExceptionTypes()
Returns an array of Type objects that represent the exceptions declared to be thrown by this Constructor object. |
8 | Type[] getGenericParameterTypes()
Returns an array of Type objects that represent the formal parameter types, in declaration order, of the method represented by this Constructor object. |
9 | Type getGenericReturnType()
Returns a Type object that represents the formal return type of the method represented by this Method object. |
10 | int getModifiers()
Returns the Java language modifiers for the method represented by this Method object, as an integer. |
11 | String getName()
Returns the name of this method, as a string. |
12 | Annotation[][] getParameterAnnotations()
Returns an array of arrays that represent the annotations on the formal parameters, in declaration order, of the method represented by this Method object. |
13 | Class<?>[] getParameterTypes()
Returns an array of Class objects that represent the formal parameter types, in declaration order, of the constructor represented by this Method object. |
14 | Class<?> getReturnType()
Returns a Class object that represents the formal return type of the method represented by this Method object. |
15 | int hashCode()
Returns a hashcode for this Constructor. |
16 | Object invoke(Object obj, Object... args)
Invokes the underlying method represented by this Method object, on the specified object with the specified parameters. |
17 | boolean isBridge()
Returns true if this method is a bridge method; returns false otherwise. |
18 | boolean isSynthetic()
Returns true if this method is a synthetic method; returns false otherwise. |
19 | boolean isVarArgs()
Returns true if this method was declared to take a variable number of arguments; returns false otherwise. |
20 | String toGenericString()
Returns a string describing this Method, including type parameters. |
21 | String toString()
Returns a string describing this Method. |
Methods inherited
This class inherits methods from the following classes −
- java.lang.reflect.AccessibleObject
- java.lang.Object
To Continue Learning Please Login