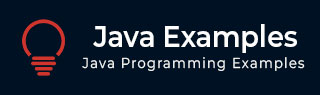
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to know the last index of a particular word in a string using Java
Problem Description
How to know the last index of a particular word in a string?
Solution
Following example demonstrates how to know the last index of a particular word in a string by using Patter.compile() method of Pattern class and matchet.find() method of Matcher class.
import java.util.regex.Matcher; import java.util.regex.Pattern; public class Main { public static void main(String args[]) { String candidateString = "This is a Java example.This is another Java example."; Pattern p = Pattern.compile("Java"); Matcher matcher = p.matcher(candidateString); matcher.find(); int nextIndex = matcher.end(); System.out.print("The last index of Java is:"); System.out.println(nextIndex); } }
Result
The above code sample will produce the following result.
The last index of Java is: 14
The following is an another sample example to know the last index of a particular word in a string.
import java.util.regex.Matcher; import java.util.regex.Pattern; public class Main { public static void main(String args[]) { String s1 = "Sairamkrishna Mammahe,Tutorialspoint india Pvt Ltd."; Pattern p1 = Pattern.compile("Tutorialspoint"); Matcher m1 = p1.matcher(s1); m1.find(); int nextIndex = m1.end(); System.out.print("The last index is:"); System.out.println(nextIndex); } }
The above code sample will produce the following result.
The last index is:36
java_regular_exp.htm
Advertisements