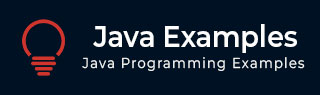
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to add image to a slide in a PPT using Java
Problem Description
How to add image to a slide in a PPT using Java.
Solution
Following is the program to add image to a slide in a PPT using java.
import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import org.apache.poi.util.IOUtils; import org.apache.poi.xslf.usermodel.XMLSlideShow; import org.apache.poi.xslf.usermodel.XSLFPictureData; import org.apache.poi.xslf.usermodel.XSLFPictureShape; import org.apache.poi.xslf.usermodel.XSLFSlide; public class AddingImageToPPT { public static void main(String args[]) throws IOException { //creating a presentation XMLSlideShow ppt = new XMLSlideShow(); //creating a slide in it XSLFSlide slide = ppt.createSlide(); //reading an image File image = new File("C:/poippt/cat.jpg"); //converting it into a byte array byte[] picture = IOUtils.toByteArray(new FileInputStream(image)); //adding the image to the presentation int idx = ppt.addPicture(picture, XSLFPictureData.PICTURE_TYPE_PNG); //creating a slide with given picture on it XSLFPictureShape pic = slide.createPicture(idx); //creating a file object File file = new File("C:/poippt/AddingimageToPPT.pptx"); FileOutputStream out = new FileOutputStream(file); //saving the changes to a file ppt.write(out); System.out.println("image added successfully"); out.close(); } }
Input
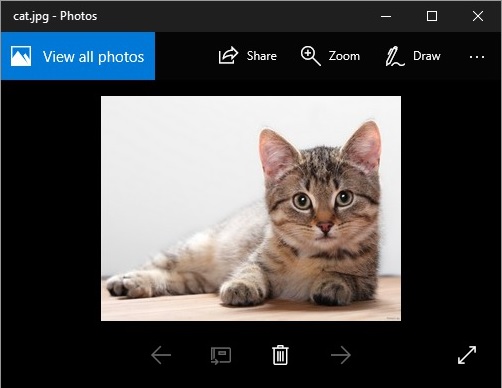
Output
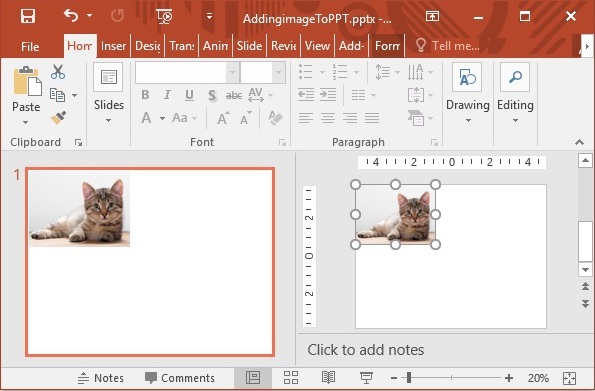
java_apache_poi_ppt
Advertisements