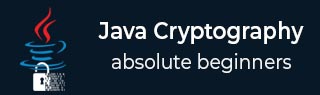
- Java Cryptography Tutorial
- Java Cryptography - Home
- Java Cryptography - Introduction
- Message Digest and MAC
- Java Cryptography - Message Digest
- Java Cryptography - Creating a MAC
- Keys and Key Store
- Java Cryptography - Keys
- Java Cryptography - Storing keys
- Java Cryptography - Retrieving keys
- Generating Keys
- KeyGenerator
- KeyPairGenerator
- Digital Signature
- Creating Signature
- Verifying Signature
- Java Cryptography Resources
- Java Cryptography - Quick Guide
- Java Cryptography - Resources
- Java Cryptography - Discussion
Java Cryptography - KeyPairGenerator
Java provides the KeyPairGenerator class. This class is used to generate pairs of public and private keys. To generate keys using the KeyPairGenerator class, follow the steps given below.
Step 1: Create a KeyPairGenerator object
The KeyPairGenerator class provides getInstance() method which accepts a String variable representing the required key-generating algorithm and returns a KeyPairGenerator object that generates keys.
Create KeyPairGenerator object using the getInstance() method as shown below.
//Creating KeyPair generator object KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance("DSA");
Step 2: Initialize the KeyPairGenerator object
The KeyPairGenerator class provides a method named initialize() this method is used to initialize the key pair generator. This method accepts an integer value representing the key size.
Initialize the KeyPairGenerator object created in the previous step using this method as shown below.
//Initializing the KeyPairGenerator keyPairGen.initialize(2048);
Step 3: Generate the KeyPairGenerator
You can generate the KeyPair using the generateKeyPair() method of the KeyPairGenerator class. Generate the key pair using this method as shown below.
//Generate the pair of keys KeyPair pair = keyPairGen.generateKeyPair();
Step 4: Get the private key/public key
You can get the private key from the generated KeyPair object using the getPrivate() method as shown below.
//Getting the private key from the key pair PrivateKey privKey = pair.getPrivate();
You can get the public key from the generated KeyPair object using the getPublic() method as shown below.
//Getting the public key from the key pair PublicKey publicKey = pair.getPublic();
Example
Following example demonstrates the key generation of the secret key using the KeyPairGenerator class of the javax.crypto package.
import java.security.KeyPair; import java.security.KeyPairGenerator; import java.security.PrivateKey; import java.security.PublicKey; public class KeyPairGenertor { public static void main(String args[]) throws Exception{ //Creating KeyPair generator object KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance("DSA"); //Initializing the KeyPairGenerator keyPairGen.initialize(2048); //Generating the pair of keys KeyPair pair = keyPairGen.generateKeyPair(); //Getting the private key from the key pair PrivateKey privKey = pair.getPrivate(); //Getting the public key from the key pair PublicKey publicKey = pair.getPublic(); System.out.println("Keys generated"); } }
Output
The above program generates the following output −
Keys generated
To Continue Learning Please Login