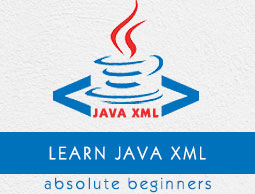
- Javax.xml.bind classes
- Home
- Binder
- DatatypeConverter
- JAXB
- JAXBContext
- JAXBElement
- JAXBElement.GlobalScope
- JAXBIntrospector
- Marshaller.Listener
- SchemaOutputResolver
- Unmarshaller.Listener
- Javax.xml.bind.util classes
- JAXBResult
- JAXBSource
- ValidationEventCollector
- Javax.xml.parsers classes
- DocumentBuilder
- DocumentBuilderFactory
- SAXParser
- SAXParserFactory
- Javax.xml.soap classes
- AttachmentPart
- MessageFactory
- MimeHeader
- MimeHeaders
- SAAJMetaFactory
- SOAPConnection
- SOAPConnectionFactory
- SOAPFactory
- SOAPMessage
- SOAPPart
- Javax.xml.validation classes
- Schema
- SchemaFactory
- TypeInfoProvider
- Validator
- ValidatorHandler
- Javax.xml.xpath classes
- XPathConstants
- XPathFactory
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
DocumentBuilder setErrorHandler() Method
Description
The Javax.xml.parsers.DocumentBuilder.setErrorHandler(ErrorHandler eh) method specifies the ErrorHandler to be used by the parser. Setting this to null will result in the underlying implementation using it's own default implementation and behavior.
Declaration
Following is the declaration for Javax.xml.parsers.DocumentBuilder.setErrorHandler() method
public abstract void setErrorHandler(ErrorHandler eh)
Parameters
eh − The ErrorHandler to be used by the parser.
Return Value
This method does not return a value.
Exception
NA
Example
For our examples to work, a xml file named Student.xml is needed in our CLASSPATH. The contents of this XML are the following −
<?xml version = "1.0" encoding = "UTF-8" standalone = "yes"?> <student id = "10"> <age>12</age> <name>Malik</name> </student>
The following example shows the usage of Javax.xml.parsers.DocumentBuilder.setErrorHandler() method.
package com.tutorialspoint; import javax.xml.parsers.DocumentBuilder; import javax.xml.parsers.DocumentBuilderFactory; import org.w3c.dom.Document; import org.w3c.dom.Element; import org.w3c.dom.NodeList; public class DocumentBuilderDemo { public static void main(String[] args) { // create a new DocumentBuilderFactory DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); try { // use the factory to create a documentbuilder DocumentBuilder builder = factory.newDocumentBuilder(); // use the default error handler builder.setErrorHandler(null); // create a new document from input stream and an empty systemId Document doc = builder.parse("Student.xml"); // get the first element Element element = doc.getDocumentElement(); // get all child nodes NodeList nodes = element.getChildNodes(); // print the text content of each child for (int i = 0; i < nodes.getLength(); i++) { System.out.println("" + nodes.item(i).getTextContent()); } } catch (Exception ex) { ex.printStackTrace(); } } }
If we compile the code and execute it, this will produce the following result −
12 Malik
javax_xml_parsers_documentbuilder.htm
Advertisements