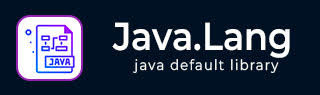
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java StringBuffer codePoints() Method
The Java StringBuffer codePoints() method is used to return the Unicode values representing the current object. Since the StringBuffer holds a sequence of values, this method will return a stream of code point values.
If this method encounters any surrogate pairs (combination of Unicode code point values in the range U+D800 to U+DBFF with other Unicode code point values in the range U+DC00 to U+DFFF) in the sequence, they are converted to their supplementary code point values using Character.toCodePoint() method and the result is passed to the integer stream.
Any other code units, including ordinary BMP (Basic Multilingual Plane) characters from U+0000 to U+FFFF, unpaired surrogates, and undefined code units, are zero-extended to int values which are then passed to the stream.
The stream binds to this sequence when the terminal stream operation starts (like when the first iteration begins). If the sequence is modified during that operation, then the result is undefined.
Syntax
Following is the syntax for the Java StringBuffer codePoints() method.
public IntStream codePoints()
Parameters
This method does not accept any parameters.
Return Value
This method returns an IntStream of Unicode code points from this sequence.
Example
When the method is invoked on a StringBuffer object, the result is the IntStream of the given sequence.
In this example, we are creating a StringBuffer object and initializing it with an input sequence of characters.
import java.lang.*; import java.util.stream.*; public class StringBuffercodePoints { public static void main(String[] args) { //create a StringBuffer object StringBuffer obj = new StringBuffer("Tutorialspoint"); IntStream s = obj.codePoints(); //print the stream generated System.out.println("The IntStream Output is: " ); s.forEach(System.out::print); } }
Output
Let us compile and run the given program, to produce the following output −
The IntStream Output is: 8411711611111410597108115112111105110116
Example
In this example, when the method is invoked on a StringBuffer object with an input sequence of digits, the result is the IntStream of the given sequence.
import java.lang.*; import java.util.stream.*; public class StringBuffercodePoints { public static void main(String[] args) { //create a StringBuffer object StringBuffer obj = new StringBuffer("123456"); IntStream s = obj.codePoints(); //print the stream generated System.out.println("The IntStream Output is: " ); s.forEach(System.out::println); } }
Output
Let us compile and run the given program, to produce the following output −
The IntStream Output is: 49 50 51 52 53 54
Example
Similarly, when the method is invoked on a StringBuffer object with an input sequence of symbols, the result is the IntStream of the given sequence.
import java.lang.*; import java.util.stream.*; public class StringBuffercodePoints { public static void main(String[] args) { //create a StringBuffer object StringBuffer obj = new StringBuffer("!@#$%^&*"); IntStream s = obj.codePoints(); //print the stream generated System.out.println("The IntStream Output is: " ); s.forEach(System.out::println); } }
Output
Let us compile and run the given program, to produce the following output −
The IntStream Output is: 33 64 35 36 37 94 38 42
Example
When the method is invoked on a StringBuffer object with an input sequence of codepoints using typecasting, the result is the IntStream of the given sequence.
import java.lang.*; import java.util.stream.*; public class StringBuffercodePoints { public static void main(String[] args) { //create a StringBuffer object StringBuffer obj = new StringBuffer(0xdc08); IntStream s = obj.codePoints(); //print the stream generated System.out.println("The IntStream Output is: "); s.forEach(System.out::print); } }
Output
Let us compile and run the given program, to produce the following output −
The IntStream Output is:
To Continue Learning Please Login