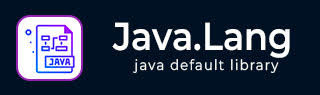
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Float toString() method
Description
The Java Float toString(float d) method returns a string representation of the float argument.The argument d is the float value to be converted.
Declaration
Following is the declaration for java.lang.Float.toString() method
public static String toString(float d)
Parameters
d − This is the float to be converted.
Return Value
This method returns a String representation of the argument.
Exception
NA
Example 1
The following example shows the usage of Float toString() method to get a string representation of a Float object. We've initialized one Float object with a positive value. Then using toString() method, we're printing the string representation value of the object.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { // returns a string value String retval = Float.toString(3.08f); System.out.println("Value = " + retval); } }
Output
Let us compile and run the above program, this will produce the following result −
Value = 3.08
Example 2
The following example shows the usage of Float toString() method to get a string representation of a Float object. We've initialized one Float object with a negative value. Then using toString() method, we're printing the string representation value of the object.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { // returns a string value String retval = Float.toString(-3.08f); System.out.println("Value = " + retval); } }
Output
Let us compile and run the above program, this will produce the following result −
Value = -3.08
Example 3
The following example shows the usage of Float toString() method to get a string representation of a Float object. We've initialized one Float object with a negative zero value. Then using toString() method, we're printing the string representation value of the object.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { // returns a string value String retval = Float.toString(-0.0f); System.out.println("Value = " + retval); } }
Output
Let us compile and run the above program, this will produce the following result −
Value = -0.0
Example 4
The following example shows the usage of Float toString() method to get a string representation of a Float object. We've initialized one Float object with a postive zero value. Then using toString() method, we're printing the string representation value of the object.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { // returns a string value String retval = Float.toString(0.0f); System.out.println("Value = " + retval); } }
Output
Let us compile and run the above program, this will produce the following result −
Value = 0.0
To Continue Learning Please Login