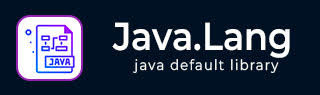
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Float toHexString() method
Description
The Java Float toHexString() method returns a hexadecimal string representation of the float argument f.Some examples can be seen here −
Floating-point Value | Hexadecimal String |
---|---|
1.0 | 0x1.0p0 |
-1.0 | -0x1.0p0 |
2.0 | 0x1.0p1 |
3.0 | 0x1.8p1 |
0.5 | 0x1.0p-1 |
0.25 | 0x1.0p-2 |
Float.MAX_VALUE | 0x1.fffffep127 |
Minimum Normal Value | 0x1.0p-126 |
Maximum Subnormal Value | 0x0.fffffep-126 |
Float.MIN_VALUE | 0x0.000002p-126 |
Declaration
Following is the declaration for java.lang.Float.toHexString() method
public static String toHexString(float f)
Parameters
f − This is the float to be converted.
Return Value
This method returns a hex string representation of the argument.
Exception
NA
Example 1
The following example shows the usage of Float toHexString(float) method to get a hex string representation of a float. We're showing multiple hex representation of positive float values along with hex representation of max float value.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { /* returns a hexadecimal string representation of the float argument */ String str = Float.toHexString(1.0f); System.out.println("Hex String = " + str); str = Float.toHexString(3.0f); System.out.println("Hex String = " + str); str = Float.toHexString(0.25f); System.out.println("Hex String = " + str); str = Float.toHexString(Float.MAX_VALUE); System.out.println("Hex String = " + str); } }
Output
Let us compile and run the above program, this will produce the following result −
Hex String = 0x1.0p0 Hex String = 0x1.8p1 Hex String = 0x1.0p-2 Hex String = 0x1.fffffep127
Example 2
The following example shows the usage of Float toHexString(float) method to get a hex string representation of a float. We're showing multiple hex representation of negative float values along with hex representation of min float value.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { /* returns a hexadecimal string representation of the float argument */ String str = Float.toHexString(-1.0f); System.out.println("Hex String = " + str); str = Float.toHexString(-3.0f); System.out.println("Hex String = " + str); str = Float.toHexString(-0.25f); System.out.println("Hex String = " + str); str = Float.toHexString(Float.MIN_VALUE); System.out.println("Hex String = " + str); } }
Output
Let us compile and run the above program, this will produce the following result −
Hex String = -0x1.0p0 Hex String = -0x1.8p1 Hex String = -0x1.0p-2 Hex String = 0x0.000002p-126
Example 3
The following example shows the usage of Float toHexString(float) method to get a hex string representation of a float. We're showing multiple hex representation of negative and positive zero values.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { /* returns a hexadecimal string representation of the float argument */ String str = Float.toHexString(-0.0f); System.out.println("Hex String = " + str); str = Float.toHexString(0.0f); System.out.println("Hex String = " + str); } }
Output
Let us compile and run the above program, this will produce the following result −
Hex String = -0x0.0p0 Hex String = 0x0.0p0
To Continue Learning Please Login