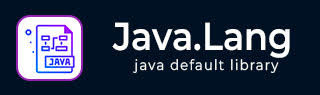
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Float parseFloat() method
Description
The Java Float parseFloat(String s) method returns a new float initialized to the value represented by the specified String, as performed by the valueOf method of class Float.
Declaration
Following is the declaration for java.lang.Float.parseFloat() method
public static float parseFloat(String s) throws NumberFormatException
Parameters
s − This is the string to be parsed.
Return Value
This method returns the float value represented by the string argument.
Exception
NumberFormatException − if the string does not contain a parsable float.
Example 1
The following example shows the usage of Float parseFloat(String) method to get a float value from a String. We've created a String with a valid float value and then using parseFloat() method, we're retrieving the float value and printing it.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { // returns the float value represented by the string argument String str = "50"; float retval = Float.parseFloat(str); System.out.println("Value = " + retval); } }
Output
Let us compile and run the above program, this will produce the following result −
Value = 50.0
Example 2
The following example shows the usage of Float parseFloat(String) method to get a float value from a String. We've created a String with an invalid float value and then using parseFloat() method, we're trying to retrieve the float value and printing it. P)rogram throws an exception as expected.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { // returns the float value represented by the string argument String str = "abc"; float retval = Float.parseFloat(str); System.out.println("Value = " + retval); } }
Output
Let us compile and run the above program, this will produce the following result −
Exception in thread "main" java.lang.NumberFormatException: For input string: "abc" at sun.misc.FloatingDecimal.readJavaFormatString(Unknown Source) at sun.misc.FloatingDecimal.parseFloat(Unknown Source) at java.lang.Float.parseFloat(Unknown Source) at com.tutorialspoint.FloatDemo.main(FloatDemo.java:9)
Example 3
The following example shows the usage of Float parseFloat(String) method to get a float value from a String. We've created a String with a valid negative float value and then using parseFloat() method, we're retrieving the float value and printing it.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { // returns the float value represented by the string argument String str = "-50"; float retval = Float.parseFloat(str); System.out.println("Value = " + retval); } }
Output
Let us compile and run the above program, this will produce the following result −
Value = -50.0
To Continue Learning Please Login