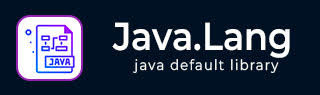
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Float isNaN(float) method
Description
The Java Float isNaN(float v) method returns true if the specified number is a Not-a-Number (NaN) value, false otherwise.The argument v is the value to be tested.
Declaration
Following is the declaration for java.lang.Float.isNan(float) method
public static boolean isNaN(float v)
Parameters
v − This is the value to be tested.
Return Value
This method returns true if the value of the argument is NaN; false otherwise.
Exception
NA
Example 1
The following example shows the usage of Float isNaN() method to check if a Float object carries a NaN value. We've initialized a Float object with an expression which result in positive infinity. Then using isNaN() method, we're checking its value.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { Float f = new Float(1.0/0.0); // returns true if NaN System.out.println(f + " = " + Float.isNaN(f)); } }
Output
Let us compile and run the above program, this will produce the following result −
Infinity = false
Example 2
The following example shows the usage of Float isNaN() method to check if a Float object carries a NaN value. We've initialized a Float object with an expression which result in negative infinity. Then using isNaN() method, we're checking its value.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { Float f = new Float(-1.0/0.0); // returns true if NaN System.out.println(f + " = " + Float.isNaN(f)); } }
Output
Let us compile and run the above program, this will produce the following result −
-Infinity = false
Example 3
The following example shows the usage of Float isNaN() method to check if a Float object carries a NaN value. We've initialized a Float object with an expression which result in NAN. Then using isNaN() method, we're checking its value.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { Float f = new Float(0.0/0.0); // returns true if NaN System.out.println(f + " = " + Float.isNaN(f)); } }
Output
Let us compile and run the above program, this will produce the following result −
NaN = true
Example 4
The following example shows the usage of Float isNaN() method to check if a Float object carries a NaN value. We've initialized a Float object with an expression which result in zero value. Then using isNaN() method, we're checking its value.
package com.tutorialspoint; public class FloatDemo { public static void main(String[] args) { Float f = new Float(0.0/1.0); // returns true if NaN System.out.println(f + " = " + Float.isNaN(f)); } }
Output
Let us compile and run the above program, this will produce the following result −
0.0 = false
To Continue Learning Please Login