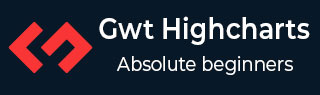
- GWT Highcharts Tutorial
- GWT Highcharts - Home
- GWT Highcharts - Overview
- Environment Setup
- Configuration Syntax
- GWT Highcharts - Line Charts
- GWT Highcharts - Area Charts
- GWT Highcharts - Bar Charts
- GWT Highcharts - Column Charts
- GWT Highcharts - Pie Charts
- GWT Highcharts - Scatter Chart
- GWT Highcharts - Dynamic Charts
- GWT Highcharts - Combinations
- GWT Highcharts - 3D Charts
- GWT Highcharts - Map Charts
- GWT Highcharts Useful Resources
- GWT Highcharts - Quick Guide
- GWT Highcharts - Useful Resources
- GWT Highcharts - Discussion
GWT Highcharts - Donut Chart
Following is an example of a Donut Chart.
We have already seen the configurations used to draw a chart in Highcharts Configuration Syntax chapter.
An example of a Donut Chart is given below.
Configurations
Let us now see the additional configurations/steps taken.
chart
Configure the chart type to be 'pie' based. chart.type decides the series type for the chart. Here, the default value is "line".
chart.setType(Type.PIE);
Example
HelloWorld.java
package com.tutorialspoint.client; import org.moxieapps.gwt.highcharts.client.Chart; import org.moxieapps.gwt.highcharts.client.Legend; import org.moxieapps.gwt.highcharts.client.Point; import org.moxieapps.gwt.highcharts.client.Series.Type; import org.moxieapps.gwt.highcharts.client.Style; import org.moxieapps.gwt.highcharts.client.ToolTip; import org.moxieapps.gwt.highcharts.client.ToolTipData; import org.moxieapps.gwt.highcharts.client.ToolTipFormatter; import org.moxieapps.gwt.highcharts.client.labels.DataLabels; import org.moxieapps.gwt.highcharts.client.labels.DataLabelsData; import org.moxieapps.gwt.highcharts.client.labels.DataLabelsFormatter; import org.moxieapps.gwt.highcharts.client.labels.PieDataLabels; import org.moxieapps.gwt.highcharts.client.labels.Labels.Align; import org.moxieapps.gwt.highcharts.client.labels.XAxisLabels; import org.moxieapps.gwt.highcharts.client.plotOptions.ColumnRangePlotOptions; import org.moxieapps.gwt.highcharts.client.plotOptions.PiePlotOptions; import org.moxieapps.gwt.highcharts.client.plotOptions.PlotOptions.Cursor; import com.google.gwt.core.client.EntryPoint; import com.google.gwt.i18n.client.NumberFormat; import com.google.gwt.user.client.ui.RootPanel; public class HelloWorld implements EntryPoint { public void onModuleLoad() { final Chart chart = new Chart() .setType(Type.PIE) .setMargin(50, 0, 0, 0) .setChartTitleText("Browser market shares at a specific website") .setChartSubtitleText("Inner circle: 2008, outer circle: 2010") .setPlotBackgroundColor("none") .setPlotBorderWidth(0) .setPlotShadow(false) .setToolTip(new ToolTip() .setFormatter(new ToolTipFormatter() { @Override public String format(ToolTipData toolTipData) { return "<b>" + toolTipData.getPointName() + "</b>: " + toolTipData.getYAsDouble() + " %"; } }) ); chart.addSeries(chart.createSeries() .setName("2008") .setPlotOptions(new PiePlotOptions() .setCenter(.5, .5) .setSize(.45) .setInnerSize(.20) .setDataLabels(new DataLabels() .setEnabled(false) ) ) .setPoints(new Point[]{ new Point("Firefox", 44.2).setColor("#4572A7"), new Point("IE", 46.6).setColor("#AA4643"), new Point("Chrome", 3.1).setColor("#89A54E"), new Point("Safari", 2.7).setColor("#80699B"), new Point("Opera", 2.3).setColor("#3D96AE"), new Point("Others", 0.4).setColor("#DB843D") }) ); chart.addSeries(chart.createSeries() .setName("2010") .setPlotOptions(new PiePlotOptions() .setCenter(.5, .5) .setInnerSize(.45) .setPieDataLabels(new PieDataLabels() .setEnabled(true) .setColor("#000000") .setConnectorColor("#000000") ) ) .setPoints(new Point[]{ new Point("Firefox", 45.0).setColor("#4572A7"), new Point("IE", 26.8).setColor("#AA4643"), new Point("Chrome", 12.8).setColor("#89A54E"), new Point("Safari", 8.5).setColor("#80699B"), new Point("Opera", 6.2).setColor("#3D96AE"), new Point("Others", 0.2).setColor("#DB843D") }) ); RootPanel.get().add(chart); } }
Result
Verify the result.
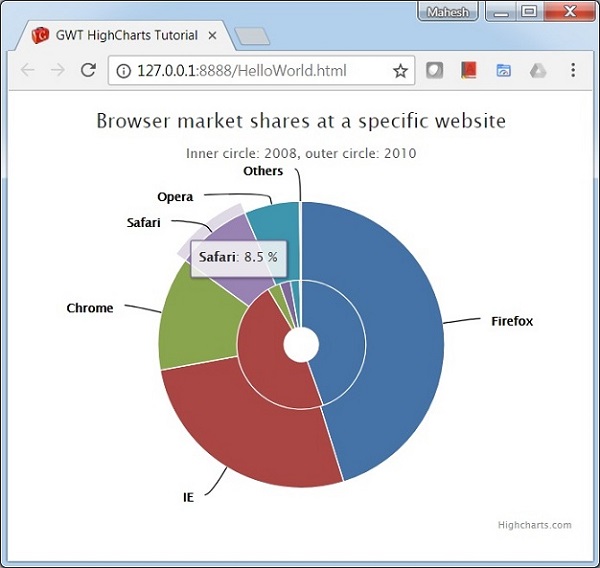
gwt_highcharts_pie_charts.htm
Advertisements
To Continue Learning Please Login