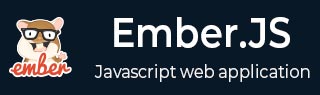
- EmberJS Tutorial
- EmberJS - Home
- EmberJS - Overview
- EmberJS - Installation
- EmberJS - Core Concepts
- Creating and Running Application
- EmberJS - Object Model
- EmberJS - Router
- EmberJS - Templates
- EmberJS - Components
- EmberJS - Models
- EmberJS - Managing Dependencies
- EmberJS - Application Concerns
- EmberJS - Configuring Ember.js
- EmberJS - Ember Inspector
- EmberJS Useful Resources
- EmberJS - Quick Guide
- EmberJS - Useful Resources
- EmberJS - Discussion
Object Model Declaring the Observer
You can define the inline observers by using the Ember.observer method without the prototype extensions.
Following is the syntax to define the inline observers using the Ember.observer method.
App.ClassName = Ember.Object.extend ({ ComputedPropertyName: Ember.observer('ComputedPropertyNames', function() { //do the stuff }) });
Outside of Class Definitions
Add the observers to an object outside of a class definition by using an addObserver() method.
The syntax can be specified as shown below −
ClassName.addObserver('ComputedPropertyNames', function() { //do the stuff });
Example
The following example specifies the inline observers by using the Ember.observer method −
import Ember from 'ember'; export default function() { var Person = Ember.Object.extend ({ Name: null, //Defining the Details1 and Details2 computed property function Details1: Ember.computed('Name', function() { //get the Name value var Name = this.get('Name'); //return the Name value return Name; }), Details2: Ember.observer('Details1', function() {}) }); //initializing the Person details var person = Person.create ({ Name: 'Steve', }); person.set('Name', 'Jhon'); document.write('Name is Changed To: ' + person.get('Details1')); }
Now open the app.js file and add the following line at the top of the file −
import outsideclassdefinitions from './outsideclassdefinitions';
Where, outsideclassdefinitions is a name of the file specified as "outsideclassdefinitions.js" and created under the "app" folder.
Next call the inherited "outsideclassdefinitions" at the bottom, before the export. It executes the outsideclassdefinitions function which is created in the outsideclassdefinitions.js file −
outsideclassdefinitions();
Output
Run the ember server and you will receive the following output −

To Continue Learning Please Login