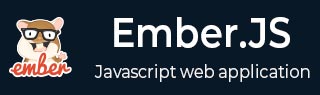
- EmberJS Tutorial
- EmberJS - Home
- EmberJS - Overview
- EmberJS - Installation
- EmberJS - Core Concepts
- Creating and Running Application
- EmberJS - Object Model
- EmberJS - Router
- EmberJS - Templates
- EmberJS - Components
- EmberJS - Models
- EmberJS - Managing Dependencies
- EmberJS - Application Concerns
- EmberJS - Configuring Ember.js
- EmberJS - Ember Inspector
- EmberJS Useful Resources
- EmberJS - Quick Guide
- EmberJS - Useful Resources
- EmberJS - Discussion
EmberJS - Object Model Chaining Computed Properties
The chaining computed property is used to aggregate with one or more predefined computed properties under a single property.
Syntax
var ClassName = Ember.Object.extend ({ NameOfComputedProperty1: Ember.computed(function() { return VariableName; }), NameOfComputedProperty2: Ember.computed(function() { return VariableName; }); });
Example
The following example shows how to use the computed properties as values to create new computed properties −
import Ember from 'ember'; export default function() { var Person = Ember.Object.extend ({ firstName: null, lastName: null, age: null, mobno: null, //Defining the Details1 and Details2 computed property function Details1: Ember.computed('firstName', 'lastName', function() { return this.get('firstName') + ' ' + this.get('lastName'); }), Details2: Ember.computed('age', 'mobno', function() { return 'Name: ' + this.get('Details1') + '<br>' + ' Age: ' + this.get('age') + '<br>' + ' Mob No: ' + this.get('mobno'); }), }); var person_details = Person.create ({ //initializing the values for variables firstName: 'Jhon', lastName: 'Smith', age: 26, mobno: '1234512345' }); document.write("<h2>Details of the Person: <br></h2>"); //displaying the values by get() method document.write(person_details.get('Details2')); }
Now open the app.js file and add the following line at the top of the file −
import chainingcomputedproperties from './chainingcomputedproperties';
Where, chainingcomputedproperties is a name of the file specified as "chainingcomputedproperties.js" and created under the "app" folder.
Now, call the inherited "chainingcomputedproperties" at the bottom, before the export. It executes the chainingcomputedproperties function which is created in the chainingcomputedproperties.js file −
chainingcomputedproperties();
Output
Run the ember server and you will receive the following output −

emberjs_object_model.htm
Advertisements
To Continue Learning Please Login