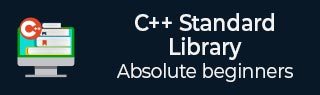
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Queue Library - queue() Function
Description
The C++ default constructor std::queue::queue() constructs queue object. If cntr argument is passed to constructor, copy of argument is assigned to container otherwise empty queue object is created.
Declaration
Following is the declaration for std::queue::queue() constructor form std::queue header.
C++98
explicit queue (const container_type& ctnr = container_type());
Parameters
ctnr − Container type which is second parameter of class template.
Return value
Constructor never returns value.
Time complexity
Linear if container of non-zero size is passed as argument otherwise constant.
Example
The following example shows the usage of std::queue::queue() constructor.
#include <iostream> #include <queue> using namespace std; int main(void) { deque<int> d(5, 100); queue<int>q1; queue<int> q2(d); cout << "Size of q1 = " << q1.size() << endl; cout << "Size of q2 = " << q2.size() << endl; return 0; }
Let us compile and run the above program, this will produce the following result −
Size of q1 = 0 Size of q2 = 5
queue.htm
Advertisements