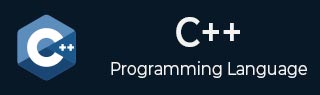
- C++ Basics
- C++ Home
- C++ Overview
- C++ Environment Setup
- C++ Basic Syntax
- C++ Comments
- C++ Data Types
- C++ Variable Types
- C++ Variable Scope
- C++ Constants/Literals
- C++ Modifier Types
- C++ Storage Classes
- C++ Operators
- C++ Loop Types
- C++ Decision Making
- C++ Functions
- C++ Numbers
- C++ Arrays
- C++ Strings
- C++ Pointers
- C++ References
- C++ Date & Time
- C++ Basic Input/Output
- C++ Data Structures
- C++ Object Oriented
- C++ Classes & Objects
- C++ Inheritance
- C++ Overloading
- C++ Polymorphism
- C++ Abstraction
- C++ Encapsulation
- C++ Interfaces
- C++ Advanced
- C++ Files and Streams
- C++ Exception Handling
- C++ Dynamic Memory
- C++ Namespaces
- C++ Templates
- C++ Preprocessor
- C++ Signal Handling
- C++ Multithreading
- C++ Web Programming
C++ Mock Test
This section presents you various set of Mock Tests related to C++ Framework. You can download these sample mock tests at your local machine and solve offline at your convenience. Every mock test is supplied with a mock test key to let you verify the final score and grade yourself.
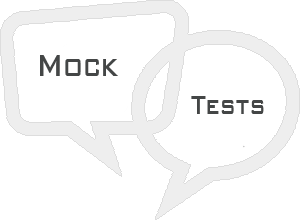
C++ Mock Test III
Q 1 - Choose the option not applicable for the constructor.
Answer : C
Explaination
A constructor can’t be overridden.
Answer : B
Explaination
An array never is passed with call by value mechanism
Q 3 - A C++ program statements can be commented using
Answer : D
Explaination
Both styles of commenting is available in C++.
Q 4 - HAS-A relationship between the classes is shown through.
Answer : B
Explaination
A class containing anther class object as its member is called as container class and exhibits HAS A relationship.
Q 5 - What is a generic class.
Answer : B
Explaination
Defining a templated class is defining a generic class. Hence functionality of the class is generalized for several types, if applicable.
Q 7 - Which type of data file is analogous to an audio cassette tape?
Answer : B
Explaination
As the access is linear.
Q 8 - i) single file can be opened by several streams simultaneously.
ii) several files simultaneously can be opened by a single stream
Answer : C
Explaination
Q 9 - With respective to streams >> (operator) is called as
Answer : B
Explaination
It extracts the data from stream into variables.
Q 10 - (i) ‘ios’ is the base class of ‘istream’
(ii) All the files are classified into only 2 types. (1) Text Files (2) Binary Files.
Answer : C
Explaination
Q 11 - An exception is __
Answer : A
Explaination
When the program is in execution phase the possible unavoidable error is called as an exception.
Q 12 - i) Exception handling technically provides multi branching.
ii) Exception handling can be mimicked using ‘goto’ construct.
Answer : A
Explaination
goto just does the unconditional branching.
Q 13 - What is the output of the following program?
#include<iostream> using namespace std; main() { const int a = 5; a++; cout<<a; }
Answer : D
Explaination
Compile error - constant variable cannot be modified.
#include<iostream> using namespace std; main() { const int a = 5; a++; cout<<a; }
Q 14 - What is the output of the following program?
#include<iostream> using namespace std; main() { char s[] = "hello", t[] = "hello"; if(s==t) cout<<"eqaul strings"; }
Answer : C
Explaination
No output, as we are comparing both base addresses and are not same.
#include<iostream> using namespace std; main() { char s[] = "hello", t[] = "hello"; if(s==t) cout<<"eqaul strings"; }
Q 15 - What is the outpout of the following program?
#include<iostream> using namespace std; main() { enum { india, is = 7, GREAT }; cout<<india<<" "<<GREAT; }
Answer : C
Explaination
0 8, enums gives the sequence starting with 0. If assigned with a value the sequence continues from the assigned value.
#include<iostream> using namespace std; main() { enum { india, is = 7, GREAT }; cout<<india<<" "<<GREAT; }
Q 16 - What is the output of the following program?
#include<iostream> using namespace std; main() { short unsigned int i = 0; cout<<i--; }
Answer : A
Explaination
0, with post decrement operator value of the variable will be considered as the expression’s value and later gets decremented
#include<iostream> using namespace std; main() { short unsigned int i = 0; cout<<i--; }
Q 17 - What is the output of the following program?
#include<iostream> using namespace std; main() { int x = 5; if(x==5) { if(x==5) break; cout<<"Hello"; } cout<<”Hi”; }
Answer : A
Explaination
compile error, keyword break can appear only within loop/switch statement.
#include<iostream> using namespace std; main() { int x = 5; if(x==5) { if(x==5) break; cout<<"Hello"; } cout<<”Hi”; }
Q 18 - What is the output of the following program?
#include<iostream> using namespace std; void f() { static int i; ++i; cout<<i<<" "; } main() { f(); f(); f(); }
Answer : D
Explaination
1 2 3, A static local variables retains its value between the function calls and the default value is 0.
#include<iostream> using namespace std; void f() { static int i; ++i; cout<<i<<" "; } main() { f(); f(); f(); }
Q 19 - What is the output of the following program?
#include<iostream> using namespace std; void f() { cout<<"Hello"<<endl; } main() { }
B - Error, as the function is not called.
C - Error, as the function is defined without its declaration
Answer : A
Explaination
No output, apart from the option (a) rest of the comments against the options are invalid
#include<iostream> using namespace std; void f() { cout<<"Hello"<<endl; } main() { }
Q 20 - What is the output of the following program?
#include <iostream> using namespace std; int main () { // local variable declaration: int x = 1; switch(x) { case 1 : cout << "Hi!" << endl; break; default : cout << "Hello!" << endl; } }
Answer : B
Explaination
Hi, control reaches default-case after comparing the rest of case constants.
#include <iostream> using namespace std; int main () { // local variable declaration: int x = 1; switch(x) { case 1 : cout << "Hi!" << endl; break; default : cout << "Hello!" << endl; } }
Q 21 - What is the output of the following program?
#include<iostream> using namespace std; void swap(int m, int n) { int x = m; m = n; n = x; } main() { int x = 5, y = 3; swap(x,y); cout<<x<<" "<<y; }
Answer : B
Explaination
5 3, call by value mechanism can’t alter actual arguments.
#include<iostream> using namespace std; void swap(int m, int n) { int x = m; m = n; n = x; } main() { int x = 5, y = 3; swap(x,y); cout<<x<<" "<<y; }
Q 22 - What will be the output of the following program?
#include<iostream> #include<string.h> using namespace std; main() { cout<<strcmp("strcmp()","strcmp()"); }
Answer : A
Explaination
0, strcmp return 0 if both the strings are equal
#include<iostream> #include<string.h> using namespace std; main() { cout<<strcmp("strcmp()","strcmp()"); }
Q 23 - What is the output of the following program?
#include<iostream> using namespace std; main() { int r, x = 2; float y = 5; r = y%x; cout<<r; }
Answer : D
Explaination
Answer Compile Error, It is invalid that either of the operands for the modulus operator (%) is a real number.
#include<iostream> using namespace std; main() { int r, x = 2; float y = 5; r = y%x; cout<<r; }
Q 24 - What is the size of the following union definition?
#include<iostream> using namespace std; main() { union abc { char a, b, c, d, e, f, g, h; int i; }; cout<<sizeof(abc); }
Answer : C
Explaination
union size is biggest element size of it. All the elements of the union share common memory.
#include<iostream> using namespace std; main() { union abc { char a, b, c, d, e, f, g, h; int i; }; cout<<sizeof(abc); }
Answer : D
Explaination
The size of ‘int’ depends upon the complier i.e. whether it is a 16 bit or 32 bit.
Answer Sheet
Question Number | Answer Key |
---|---|
1 | C |
2 | B |
3 | D |
4 | B |
5 | B |
6 | A |
7 | B |
8 | C |
9 | B |
10 | C |
11 | A |
12 | A |
13 | D |
14 | C |
15 | C |
16 | A |
17 | A |
18 | D |
19 | A |
20 | B |
21 | B |
22 | A |
23 | D |
24 | C |
25 | D |
To Continue Learning Please Login