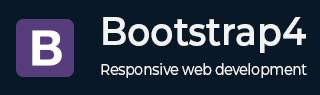
- Bootstrap 4 Tutorial
- Bootstrap 4 - Home
- Bootstrap 4 - Overview
- Bootstrap 4 - Environment Setup
- Bootstrap 4 - Layout
- Bootstrap 4 - Grid System
- Bootstrap 4 - Content
- Bootstrap 4 - Components
- Bootstrap 4 - Utilities
- Differences Between Bootstrap 3 and 4
- Bootstrap 4 Useful Resources
- Bootstrap 4 - Quick Guide
- Bootstrap 4 - Useful Resources
- Bootstrap 4 - Discussion
Bootstrap 4 - Scrollspy
Description
Scrollspy is used to indicate currently active link in the menu, based on scroll position.
Basic Scrollspy
Scrollspy uses data-spy = "scroll" attribute, which can be used as the scrollable area (often this is the
element) and add the data-target attribute with the ID or class of the parent element of any Bootstrap .navbar component to indicate navbar is connected with scrollable area and nav links will be highlighted. The optional data-offset attribute defines number of pixels which calculates the position of scroll from top.The following example shows the usage of scrollspy −
Example
<html lang = "en"> <head> <!-- Meta tags --> <meta charset = "utf-8"> <meta name = "viewport" content = "width = device-width, initial-scale = 1, shrink-to-fit = no"> <!-- Bootstrap CSS --> <link rel = "stylesheet" href = "https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css" integrity = "sha384-MCw98/SFnGE8fJT3GXwEOngsV7Zt27NXFoaoApmYm81iuXoPkFOJwJ8ERdknLPMO" crossorigin = "anonymous"> <title>Bootstrap 4 Example</title> <style> #Scrollspy { position: relative; overflow-y: scroll; height: 300px; } #Scrollspynested { height: 200px; overflow-y: scroll; } #container { overflow: hidden; } </style> </head> <body> <div class = "container-fluid"> <h2>Scrollspy</h2> <div id = "Scrollspy" data-spy = "scroll" data-target = ".navbar" data-offset = "50"> <nav class = "navbar navbar-expand-sm bg-dark navbar-dark fixed-top"> <ul class = "navbar-nav"> <li class = "nav-item"> <a class = "nav-link" href = "#section1">Section 1</a> </li> <li class = "nav-item"> <a class = "nav-link" href = "#section2">Section 2</a> </li> <li class = "nav-item"> <a class = "nav-link" href = "#section3">Section 3</a> </li> <li class = "nav-item dropdown"> <a class = "nav-link dropdown-toggle" href = "#" id = "navbardrop" data-toggle = "dropdown"> Section 4 </a> <div class = "dropdown-menu"> <a class = "dropdown-item" href = "#section41">Link 1</a> <a class = "dropdown-item" href = "#section42">Link 2</a> </div> </li> </ul> </nav> <div id = "section1" class = "container-fluid" style = "padding-top:70px;padding-bottom:70px"> <h1>This is Section 1</h1> <p>Cotent for section #1</p> </div> <div id = "section2" class = "container-fluid" style = "padding-top:70px;padding-bottom:70px"> <h1>This is Section 2</h1> <p>Cotent for section #2</p> </div> <div id = "section3" class = "container-fluid" style = "padding-top:70px;padding-bottom:70px"> <h1>This is Section 3</h1> <p>Cotent for section #3</p> </div> <div id = "section41" class = "container-fluid" style = "padding-top:70px;padding-bottom:70px"> <h1>This is Section 4 Submenu 1</h1> <p>Cotent for section #4.1</p> </div> <div id = "section42" class = "container-fluid" style = "padding-top:70px;padding-bottom:70px"> <h1>This is Section 4 Submenu 2</h1> <p>Cotent for section #4.2</p> </div> </div> </div> <!-- jQuery library --> <script src = "https://code.jquery.com/jquery-3.2.1.slim.min.js" integrity = "sha384-KJ3o2DKtIkvYIK3UENzmM7KCkRr/rE9/Qpg6aAZGJwFDMVNA/GpGFF93hXpG5KkN" crossorigin = "anonymous"> </script> <!-- Popper --> <script src = "https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js" integrity = "sha384-ApNbgh9B+Y1QKtv3Rn7W3mgPxhU9K/ScQsAP7hUibX39j7fakFPskvXusvfa0b4Q" crossorigin = "anonymous"> </script> <!-- Latest compiled and minified Bootstrap JavaScript --> <script src = "https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js" integrity = "sha384-JZR6Spejh4U02d8jOt6vLEHfe/JQGiRRSQQxSfFWpi1MquVdAyjUar5+76PVCmYl" crossorigin = "anonymous"> </script> </body> </html>
It will produce the following result −
Output
Scrollspy Vertical Menu
You can use the navigation pills as vertical menu by using scrollspy as shown in the below example −
Example
<html lang = "en"> <head> <!-- Meta tags --> <meta charset = "utf-8"> <meta name = "viewport" content = "width = device-width, initial-scale = 1, shrink-to-fit = no"> <!-- Bootstrap CSS --> <link rel = "stylesheet" href = "https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css" integrity = "sha384-MCw98/SFnGE8fJT3GXwEOngsV7Zt27NXFoaoApmYm81iuXoPkFOJwJ8ERdknLPMO" crossorigin = "anonymous"> <title>Bootstrap 4 Example</title> <style> body { position: relative; } ul.nav-pills { top: 50px; position: fixed; } div.col-8 div { height: 500px; } </style> </head> <body data-spy = "scroll" data-target = "#myScrollspy" data-offset = "1"> <h2>Scrollspy Vertical Menu</h2> <div class = "container-fluid"> <div class = "row"> <nav class = "col-sm-3 col-4" id = "myScrollspy"> <ul class = "nav nav-pills flex-column"> <li class = "nav-item"> <a class = "nav-link active" href = "#section1">Section 1</a> </li> <li class = "nav-item"> <a class = "nav-link" href = "#section2">Section 2</a> </li> <li class = "nav-item dropdown"> <a class = "nav-link dropdown-toggle" data-toggle = "dropdown" href = "#">Section 3</a> <div class = "dropdown-menu"> <a class = "dropdown-item" href = "#section31">dropdown-item 1</a> <a class = "dropdown-item" href = "#section32">dropdown-item 2</a> </div> </li> <li class = "nav-item"> <a class = "nav-link" href = "#section4">Section 4</a> </li> </ul> </nav> <div class = "col-sm-9 col-8"> <div id = "section1" > <h1>This is Section 1</h1> <p>Content for section #1</p> </div> <div id = "section2" > <h1>This is Section 2</h1> <p>Content for section #2</p> </div> <div id = "section31" > <h1>This is Section 3.1</h1> <p>Content for section #3.1</p> </div> <div id = "section32" > <h1>This is Section 3.2</h1> <p>Content for section #3.2</p> </div> <div id = "section4" > <h1>This is Section 4</h1> <p>Content for section #4</p> </div> </div> </div> </div> <!-- jQuery library --> <script src = "https://code.jquery.com/jquery-3.2.1.slim.min.js" integrity = "sha384-KJ3o2DKtIkvYIK3UENzmM7KCkRr/rE9/Qpg6aAZGJwFDMVNA/GpGFF93hXpG5KkN" crossorigin = "anonymous"> </script> <!-- Popper --> <script src = "https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js" integrity = "sha384-ApNbgh9B+Y1QKtv3Rn7W3mgPxhU9K/ScQsAP7hUibX39j7fakFPskvXusvfa0b4Q" crossorigin = "anonymous"> </script> <!-- Latest compiled and minified Bootstrap JavaScript --> <script src = "https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js" integrity = "sha384-JZR6Spejh4U02d8jOt6vLEHfe/JQGiRRSQQxSfFWpi1MquVdAyjUar5+76PVCmYl" crossorigin = "anonymous"> </script> </body> </html>
It will produce the following result −
Output
bootstrap4_components.htm
Advertisements
To Continue Learning Please Login