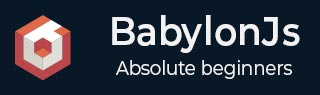
- BabylonJS Tutorial
- BabylonJS - Home
- BabylonJS - Introduction
- BabylonJS - Environment Setup
- BabylonJS - Overview
- BabylonJS - Basic Elements
- BabylonJS - Materials
- BabylonJS - Animations
- BabylonJS - Cameras
- BabylonJS - Lights
- BabylonJS - Parametric Shapes
- BabylonJS - Mesh
- VectorPosition and Rotation
- BabylonJS - Decals
- BabylonJS - Curve3
- BabylonJS - Dynamic Texture
- BabylonJS - Parallax Mapping
- BabylonJS - Lens Flares
- BabylonJS - Create ScreenShot
- BabylonJS - Reflection Probes
- Standard Rendering Pipeline
- BabylonJS - ShaderMaterial
- BabylonJS - Bones and Skeletons
- BabylonJS - Physics Engine
- BabylonJS - Playing Sounds & Music
- BabylonJS Useful Resources
- BabylonJS - Quick Guide
- BabylonJS - Useful Resources
- BabylonJS - Discussion
BabylonJS - Sphere
In this section, we will learn how to add Sphere to the scene we created.
Syntax
var sphere = BABYLON.Mesh.CreateSphere("sphere", 10.0, 10.0, scene, false, BABYLON.Mesh.DEFAULTSIDE);
Parameters
following parameters to add Sphere −
Name − This is the Name of the sphere.
Segments − This shows the number of segments.
Size − This is the size of the sphere.
Scene − This is the scene to be attached.
Boolean − This is updatable if the mesh needs to be modified later.
BABYLON.Mesh.DEFAULTSIDE − This is the optional side orientation.
The last two parameters are optional.
We have already seen an example of sphere while creating the scene. Let us now glance through the creation of sphere again.
Demo - Sphere
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>MDN Games: Babylon.js demo - shapes</title> <script src = "babylon.js"></script> <style> html,body,canvas { margin: 0; padding: 0; width: 100%; height: 100%; font-size: 0; } </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3(0, 1, 0); var camera = new BABYLON.ArcRotateCamera("Camera", 1, 0.8, 10, new BABYLON.Vector3(0, 0, 0), scene); var light0 = new BABYLON.PointLight("Omni", new BABYLON.Vector3(0, 0, 10), scene); var origin = BABYLON.Mesh.CreateSphere("origin", 15, 5.0, scene); scene.activeCamera.attachControl(canvas); return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
Output
Upon execution we get following output −
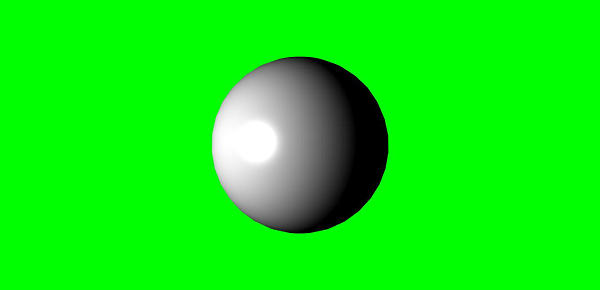
Sphere can also be created using the meshbuilder.
Syntax
Following is the syntax to create a sphere using the meshbuilder −
var sphere = BABYLON.MeshBuilder.CreateSphere("sphere", {diameter: 2, diameterX: 3}, scene);
The Properties for a Sphere
Consider the following properties for a sphere. These properties are optional.
Segments − The default value is 32.It is for the horizontal segments.
Diameter − This is the diameter of the sphere, the default value of which is 1.
DiameterX − The diameter on X-axis overwrites the diameter property. If not specified, it uses the diameter property.
DiameterY − The diameter on Y-axis overwrites the diameter property. If not specified, it uses the diameter property.
DiameterZ − The diameter of Z-axis, overwrites the diameter property. If not specified, it uses the diameter property.
Arc − This is the ratio of the circumference (latitude) between 0 and 1.
Slice − This is the ratio of the height (longitude) between 0 and 1.
Boolean − The Boolean value is true if the mesh is updatable. By default, it is false.
SideOrientation − This refers to the side orientation.
To Continue Learning Please Login