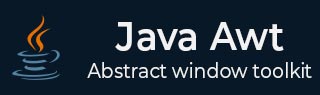
- AWT Tutorial
- AWT - Home
- AWT - Overview
- AWT - Environment
- AWT - Controls
- AWT - Event Handling
- AWT - Event Classes
- AWT - Event Listeners
- AWT - Event Adapters
- AWT - Layouts
- AWT - Containers
- AWT - Menu
- AWT - Graphics
- AWT Useful Resources
- AWT - Quick Guide
- AWT - Useful Resources
- AWT - discussion
AWT WindowListener Interface
The class which processes the WindowEvent should implement this interface.The object of that class must be registered with a component. The object can be registered using the addWindowListener() method.
Interface declaration
Following is the declaration for java.awt.event.WindowListener interface:
public interface WindowListener extends EventListener
Interface methods
S.N. | Method & Description |
---|---|
1 | void windowActivated(WindowEvent e) Invoked when the Window is set to be the active Window. |
2 | void windowClosed(WindowEvent e) Invoked when a window has been closed as the result of calling dispose on the window. |
3 | void windowClosing(WindowEvent e) Invoked when the user attempts to close the window from the window's system menu. |
4 | void windowDeactivated(WindowEvent e) Invoked when a Window is no longer the active Window. |
5 | void windowDeiconified(WindowEvent e) Invoked when a window is changed from a minimized to a normal state. |
6 | void windowIconified(WindowEvent e) Invoked when a window is changed from a normal to a minimized state. |
7 | void windowOpened(WindowEvent e) Invoked the first time a window is made visible. |
Methods inherited
This interface inherits methods from the following interfaces:
java.awt.EventListener
WindowListener Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >
AwtListenerDemo.javapackage com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtListenerDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtListenerDemo(){ prepareGUI(); } public static void main(String[] args){ AwtListenerDemo awtListenerDemo = new AwtListenerDemo(); awtListenerDemo.showWindowListenerDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showWindowListenerDemo(){ headerLabel.setText("Listener in action: WindowListener"); Button okButton = new Button("OK"); aboutFrame = new Frame(); aboutFrame.setSize(300,200);; aboutFrame.setTitle("WindowListener Demo"); aboutFrame.addWindowListener(new CustomWindowListener()); Label msgLabel = new Label("Welcome to tutorialspoint."); msgLabel.setAlignment(Label.CENTER); msgLabel.setSize(100,100); aboutFrame.add(msgLabel); aboutFrame.setVisible(true); } class CustomWindowListener implements WindowListener { public void windowOpened(WindowEvent e) { } public void windowClosing(WindowEvent e) { aboutFrame.dispose(); } public void windowClosed(WindowEvent e) { } public void windowIconified(WindowEvent e) { } public void windowDeiconified(WindowEvent e) { } public void windowActivated(WindowEvent e) { } public void windowDeactivated(WindowEvent e) { } } }
Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtListenerDemo.java
If no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtListenerDemo
Verify the following output
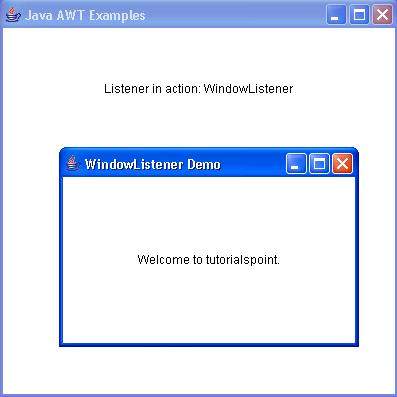