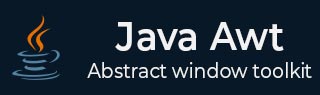
- AWT Tutorial
- AWT - Home
- AWT - Overview
- AWT - Environment
- AWT - Controls
- AWT - Event Handling
- AWT - Event Classes
- AWT - Event Listeners
- AWT - Event Adapters
- AWT - Layouts
- AWT - Containers
- AWT - Menu
- AWT - Graphics
- AWT Useful Resources
- AWT - Quick Guide
- AWT - Useful Resources
- AWT - discussion
AWT FocusListener Interface
Introduction
The interfaceFocusListener is used for receiving keyboard focus events. The class that process focus events needs to implements this interface.
Class declaration
Following is the declaration for java.awt.event.FocusListener interface:
public interface FocusListener extends EventListener
Interface methods
S.N. | Method & Description |
---|---|
1 | void focusGained(FocusEvent e) Invoked when a component gains the keyboard focus. |
2 | void focusLost(FocusEvent e) Invoked when a component loses the keyboard focus. |
Methods inherited
This class inherits methods from the following interfaces:
java.awt.event.EventListener
FocusListener Example
Create the following java program using any editor of your choice in say D:/ > AWT > com > tutorialspoint > gui >
AwtListenerDemo.javapackage com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; public class AwtListenerDemo { private Frame mainFrame; private Label headerLabel; private Label statusLabel; private Panel controlPanel; public AwtListenerDemo(){ prepareGUI(); } public static void main(String[] args){ AwtListenerDemo awtListenerDemo = new AwtListenerDemo(); awtListenerDemo.showFocusListenerDemo(); } private void prepareGUI(){ mainFrame = new Frame("Java AWT Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new Label(); headerLabel.setAlignment(Label.CENTER); statusLabel = new Label(); statusLabel.setAlignment(Label.CENTER); statusLabel.setSize(350,100); controlPanel = new Panel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showFocusListenerDemo(){ headerLabel.setText("Listener in action: FocusListener"); Button okButton = new Button("OK"); Button cancelButton = new Button("Cancel"); okButton.addFocusListener(new CustomFocusListener()); cancelButton.addFocusListener(new CustomFocusListener()); controlPanel.add(okButton); controlPanel.add(cancelButton); mainFrame.setVisible(true); } class CustomFocusListener implements FocusListener{ public void focusGained(FocusEvent e) { statusLabel.setText(statusLabel.getText() + e.getComponent().getClass().getSimpleName() + " gained focus. "); } public void focusLost(FocusEvent e) { statusLabel.setText(statusLabel.getText() + e.getComponent().getClass().getSimpleName() + " lost focus. "); } } }
Compile the program using command prompt. Go to D:/ > AWT and type the following command.
D:\AWT>javac com\tutorialspoint\gui\AwtListenerDemo.java
If no error comes that means compilation is successful. Run the program using following command.
D:\AWT>java com.tutorialspoint.gui.AwtListenerDemo
Verify the following output
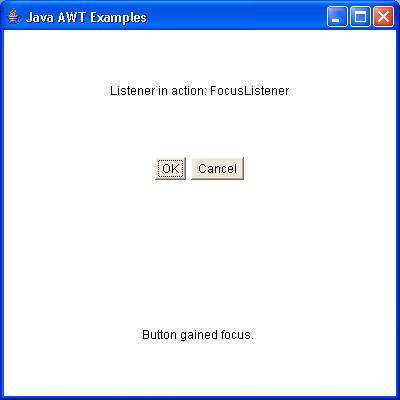