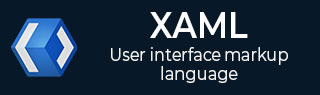
- XAML Tutorial
- XAML - Home
- XAML - Overview
- XAML - Environment Setup
- Writing XAML Aplication On MAC OS
- XAML Vs C# Code
- XAML Vs.VB.NET
- XAML - Building Blocks
- XAML - Controls
- XAML - Layouts
- XAML - Event Handling
- XAML - Data Binding
- XAML - Markup Extensions
- XAML - Dependency Properties
- XAML - Resources
- XAML - Templates
- XAML - Styles
- XAML - Triggers
- XAML - Debugging
- XAML - Custom Controls
- XAML Useful Resources
- XAML - Quick Guide
- XAML - Useful Resources
- XAML - Discussion
XAML - Slider
A Slider is a control with the help of which a user can select from a range of values by moving a Thumb control along a track. The hierarchical inheritance of the Slider class is as follows −
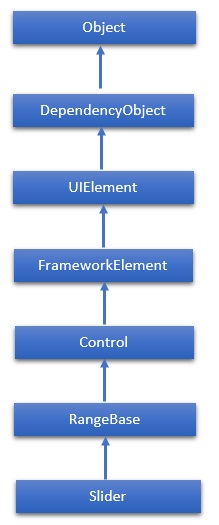
Properties
Sr.No. | Property & Description |
---|---|
1 | Header Gets or sets the content for the control's header. |
2 | HeaderProperty Identifies the Header dependency property. |
3 | HeaderTemplate Gets or sets the DataTemplate used to display the content of the control's header. |
4 | HeaderTemplateProperty Identifies the HeaderTemplate dependency property. |
5 | IntermediateValue Gets or sets the value of the Slider while the user is interacting with it, before the value is snapped to either the tick or step value. The value the Slider snaps to is specified by the SnapsTo property. |
6 | IntermediateValueProperty Identifies the IntermediateValue dependency property. |
7 | IsDirectionReversed Gets or sets a value that indicates the direction of increasing value. |
8 | IsDirectionReversedProperty Identifies the IsDirectionReversed dependency property. |
9 | IsThumbToolTipEnabled Gets or sets a value that determines whether the slider value is shown in a tool tip for the Thumb component of the Slider. |
10 | IsThumbToolTipEnabledProperty Identifies the IsThumbToolTipEnabled dependency property. |
11 | Orientation Gets or sets the orientation of a Slider. |
12 | OrientationProperty Identifies the Orientation dependency property. |
13 | StepFrequency Gets or sets the value part of a value range that steps should be created for. |
14 | StepFrequencyProperty Identifies the StepFrequency dependency property. |
15 | ThumbToolTipValueConverter Gets or sets the converter logic that converts the range value of the Slider into tool tip content. |
16 | ThumbToolTipValueConverterProperty Identifies the ThumbToolTipValueConverter dependency property. |
17 | TickFrequency Gets or sets the increment of the value range that ticks should be created for. |
18 | TickFrequencyProperty Identifies the TickFrequency dependency property. |
19 | TickPlacement Gets or sets a value that indicates where to draw tick marks in relation to the track. |
20 | TickPlacementProperty Identifies the TickPlacement dependency property. |
Events
Sr.No. | Event & Description |
---|---|
1 | ManipulationCompleted Occurs when a manipulation on the UIElement is complete. (Inherited from UIElement) |
2 | ManipulationDelta Occurs when the input device changes position during a manipulation. (Inherited from UIElement) |
3 | ManipulationInertiaStarting Occurs when the input device loses contact with the UIElement object during a manipulation and inertia begins. (Inherited from UIElement) |
4 | ManipulationStarted Occurs when an input device begins a manipulation on the UIElement. (Inherited from UIElement) |
5 | ManipulationStarting Occurs when the manipulation processor is first created. (Inherited from UIElement) |
6 | ValueChanged Occurs when the range value changes. (Inherited from RangeBase) |
Methods
Sr.No. | Method & Description |
---|---|
1 | OnManipulationCompleted Called before the ManipulationCompleted event occurs. (Inherited from Control) |
2 | OnManipulationDelta Called before the ManipulationDelta event occurs. (Inherited from Control) |
3 | OnManipulationInertiaStarting Called before the ManipulationInertiaStarting event occurs. (Inherited from Control) |
4 | OnManipulationStarted Called before the ManipulationStarted event occurs. (Inherited from Control) |
5 | OnManipulationStarting Called before the ManipulationStarting event occurs. (Inherited from Control) |
6 | OnMaximumChanged Called when the Maximum property changes. (Inherited from RangeBase) |
7 | OnMinimumChanged Called when the Minimum property changes. (Inherited from RangeBase) |
8 | OnValueChanged Fires the ValueChanged routed event. (Inherited from RangeBase) |
9 | SetBinding Attaches a binding to a FrameworkElement, using the provided binding object. (Inherited from FrameworkElement) |
10 | SetValue Sets the local value of a dependency property on a DependencyObject. (Inherited from DependencyObject) |
Example
The following example shows the usage of Slider in an XAML application. Here is the XAML code to create a Slider and text blocks with some properties and events.
<Window x:Class = "XAMLSlider.MainWindow" xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml" Title = "MainWindow" Height = "350" Width = "525"> <Grid> <StackPanel> <TextBlock Text = "Slider with ValueChanged event handler:" Margin = "10"/> <Slider x:Name = "slider2" Minimum = "0" Maximum = "100" TickFrequency = "2" TickPlacement = "BottomRight" ValueChanged = "slider2_ValueChanged" Margin = "10"/> <TextBlock x:Name = "textBlock1" Margin = "10" Text = "Current value: 0" /> </StackPanel> </Grid> </Window>
Here is the implementation in C# for ValueChanged event −
using System; using System.Windows; using System.Windows.Controls; namespace XAMLSlider { /// <summary> /// Interaction logic for MainWindow.xaml /// </summary> public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); } //private void slider2_ValueChanged(object sender,RangeBaseValueChangedEventArgs e) //{ // string msg = String.Format("Current value: {0}", e.NewValue); // this.textBlock1.Text = msg; //} private void slider2_ValueChanged(object sender,RoutedPropertyChangedEventArgs<double> e) { int val = Convert.ToInt32(e.NewValue); string msg = String.Format("Current value: {0}", val); this.textBlock1.Text = msg; } } }
When you compile and execute the above code, it will produce the following output −
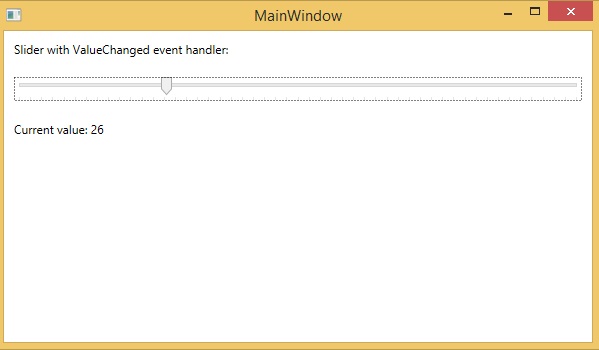
We recommend you to execute the above example code and experiment with some other properties and events.
To Continue Learning Please Login