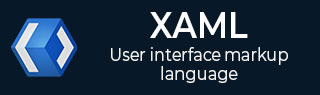
- XAML Tutorial
- XAML - Home
- XAML - Overview
- XAML - Environment Setup
- Writing XAML Aplication On MAC OS
- XAML Vs C# Code
- XAML Vs.VB.NET
- XAML - Building Blocks
- XAML - Controls
- XAML - Layouts
- XAML - Event Handling
- XAML - Data Binding
- XAML - Markup Extensions
- XAML - Dependency Properties
- XAML - Resources
- XAML - Templates
- XAML - Styles
- XAML - Triggers
- XAML - Debugging
- XAML - Custom Controls
- XAML Useful Resources
- XAML - Quick Guide
- XAML - Useful Resources
- XAML - Discussion
XAML - Dialog Box
All standalone applications have a main window that exposes some functionality and displays some data over which the application operates through its GUI. An application may also display additional windows to do the following −
- To display some specific information to users.
- To gather useful information from users.
- To both display and gather important information.
Example
Let’s have a look at the following example. On the main window, there is a button and a textbox. When the user clicks this button, it opens another dialog box with Yes, No, and Cancel buttons and displays a message on it that prompts the user to click a button.
When the user clicks a button, then the current dialog box gets closed and shows a textbox with the information "which button has been clicked".
Here is the XAML code to create and initialize a button and a textbox with some properties −
<Window x:Class = "XAMLDialog.MainWindow" xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml" Title = "MainWindow" Height = "350" Width = "604"> <Grid> <Button Height = "23" Margin = "100" Name = "ShowMessageBox" VerticalAlignment = "Top" Click = "ShowMessageBox_Click">Show Message Box</Button> <TextBox Height = "23" HorizontalAlignment = "Left" Margin = "181,167,0,0" Name = "textBox1" VerticalAlignment = "Top" Width = "120" /> </Grid> </Window>
Given below is the C# code to implement a button click event.
using System; using System.Windows; using System.Windows.Controls; namespace XAMLDialog { /// <summary> /// Interaction logic for MainWindow.xaml /// </summary> public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); } private void ShowMessageBox_Click(object sender, RoutedEventArgs e) { string msgtext = "Click any button"; string txt = "My Title"; MessageBoxButton button = MessageBoxButton.YesNoCancel; MessageBoxResult result = MessageBox.Show(msgtext, txt, button); switch (result) { case MessageBoxResult.Yes: textBox1.Text = "Yes"; break; case MessageBoxResult.No: textBox1.Text = "No"; break; case MessageBoxResult.Cancel: textBox1.Text = "Cancel"; break; } } } }
When you compile and execute the above code, it will produce the following output −
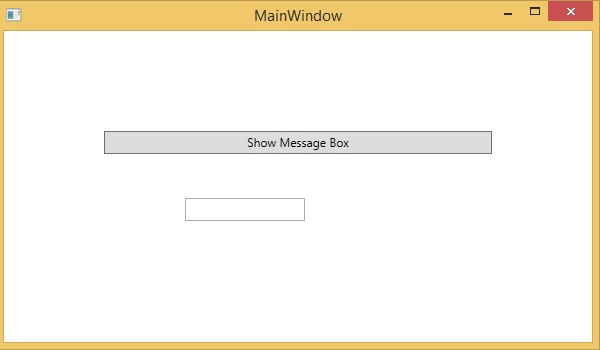
When you click on the button, it displays another dialog box as shown below that prompts the user to click a button. Now, click the Yes button.
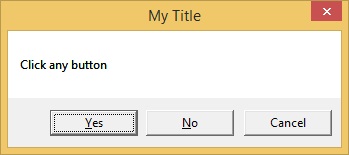
It updates the textbox with the button content.
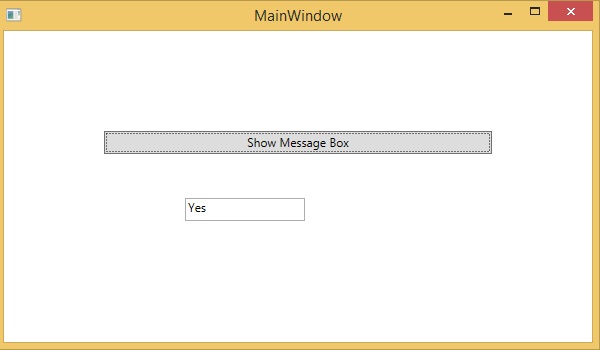