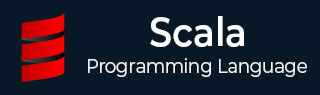
- Scala Tutorial
- Scala - Home
- Scala - Overview
- Scala - Environment Setup
- Scala - Basic Syntax
- Scala - Data Types
- Scala - Variables
- Scala - Classes & Objects
- Scala - Access Modifiers
- Scala - Operators
- Scala - IF ELSE
- Scala - Loop Statements
- Scala - Functions
- Scala - Closures
- Scala - Strings
- Scala - Arrays
- Scala - Collections
- Scala - Traits
- Scala - Pattern Matching
- Scala - Regular Expressions
- Scala - Exception Handling
- Scala - Extractors
- Scala - Files I/O
- Scala Useful Resources
- Scala - Quick Guide
- Scala - Useful Resources
- Scala - Discussion
Scala - Pattern Matching
Pattern matching is the second most widely used feature of Scala, after function values and closures. Scala provides great support for pattern matching, in processing the messages.
A pattern match includes a sequence of alternatives, each starting with the keyword case. Each alternative includes a pattern and one or more expressions, which will be evaluated if the pattern matches. An arrow symbol => separates the pattern from the expressions.
Try the following example program, which shows how to match against an integer value.
Example
object Demo { def main(args: Array[String]) { println(matchTest(3)) } def matchTest(x: Int): String = x match { case 1 => "one" case 2 => "two" case _ => "many" } }
Save the above program in Demo.scala. The following commands are used to compile and execute this program.
Command
\>scalac Demo.scala \>scala Demo
Output
many
The block with the case statements defines a function, which maps integers to strings. The match keyword provides a convenient way of applying a function (like the pattern matching function above) to an object.
Try the following example program, which matches a value against patterns of different types.
Example
object Demo { def main(args: Array[String]) { println(matchTest("two")) println(matchTest("test")) println(matchTest(1)) } def matchTest(x: Any): Any = x match { case 1 => "one" case "two" => 2 case y: Int => "scala.Int" case _ => "many" } }
Save the above program in Demo.scala. The following commands are used to compile and execute this program.
Command
\>scalac Demo.scala \>scala Demo
Output
2 many one
Matching using Case Classes
The case classes are special classes that are used in pattern matching with case expressions. Syntactically, these are standard classes with a special modifier: case.
Try the following, it is a simple pattern matching example using case class.
Example
object Demo { def main(args: Array[String]) { val alice = new Person("Alice", 25) val bob = new Person("Bob", 32) val charlie = new Person("Charlie", 32) for (person <- List(alice, bob, charlie)) { person match { case Person("Alice", 25) => println("Hi Alice!") case Person("Bob", 32) => println("Hi Bob!") case Person(name, age) => println( "Age: " + age + " year, name: " + name + "?") } } } case class Person(name: String, age: Int) }
Save the above program in Demo.scala. The following commands are used to compile and execute this program.
Command
\>scalac Demo.scala \>scala Demo
Output
Hi Alice! Hi Bob! Age: 32 year, name: Charlie?
Adding the case keyword causes the compiler to add a number of useful features automatically. The keyword suggests an association with case expressions in pattern matching.
First, the compiler automatically converts the constructor arguments into immutable fields (vals). The val keyword is optional. If you want mutable fields, use the var keyword. So, our constructor argument lists are now shorter.
Second, the compiler automatically implements equals, hashCode, and toString methods to the class, which use the fields specified as constructor arguments. So, we no longer need our own toString() methods.
Finally, also, the body of Person class becomes empty because there are no methods that we need to define!