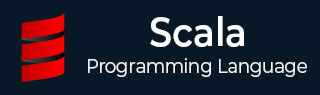
- Scala Tutorial
- Scala - Home
- Scala - Overview
- Scala - Environment Setup
- Scala - Basic Syntax
- Scala - Data Types
- Scala - Variables
- Scala - Classes & Objects
- Scala - Access Modifiers
- Scala - Operators
- Scala - IF ELSE
- Scala - Loop Statements
- Scala - Functions
- Scala - Closures
- Scala - Strings
- Scala - Arrays
- Scala - Collections
- Scala - Traits
- Scala - Pattern Matching
- Scala - Regular Expressions
- Scala - Exception Handling
- Scala - Extractors
- Scala - Files I/O
- Scala Useful Resources
- Scala - Quick Guide
- Scala - Useful Resources
- Scala - Discussion
Scala - Exception Handling
Scala's exceptions work like exceptions in many other languages like Java. Instead of returning a value in the normal way, a method can terminate by throwing an exception. However, Scala doesn't actually have checked exceptions.
When you want to handle exceptions, you use a try{...}catch{...} block like you would in Java except that the catch block uses matching to identify and handle the exceptions.
Throwing Exceptions
Throwing an exception looks the same as in Java. You create an exception object and then you throw it with the throw keyword as follows.
throw new IllegalArgumentException
Catching Exceptions
Scala allows you to try/catch any exception in a single block and then perform pattern matching against it using case blocks. Try the following example program to handle exception.
Example
import java.io.FileReader import java.io.FileNotFoundException import java.io.IOException object Demo { def main(args: Array[String]) { try { val f = new FileReader("input.txt") } catch { case ex: FileNotFoundException =>{ println("Missing file exception") } case ex: IOException => { println("IO Exception") } } } }
Save the above program in Demo.scala. The following commands are used to compile and execute this program.
Command
\>scalac Demo.scala \>scala Demo
Output
Missing file exception
The behavior of this try-catch expression is the same as in other languages with exceptions. The body is executed, and if it throws an exception, each catch clause is tried in turn.
The finally Clause
You can wrap an expression with a finally clause if you want to cause some code to execute no matter how the expression terminates. Try the following program.
Example
import java.io.FileReader import java.io.FileNotFoundException import java.io.IOException object Demo { def main(args: Array[String]) { try { val f = new FileReader("input.txt") } catch { case ex: FileNotFoundException => { println("Missing file exception") } case ex: IOException => { println("IO Exception") } } finally { println("Exiting finally...") } } }
Save the above program in Demo.scala. The following commands are used to compile and execute this program.
Command
\>scalac Demo.scala \>scala Demo
Output
Missing file exception Exiting finally...