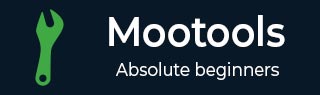
- MooTools Tutorial
- MooTools - Home
- MooTools - Introduction
- MooTools - Installation
- MooTools - Program Structure
- MooTools - Selectors
- MooTools - Using Arrays
- MooTools - Functions
- MooTools - Event Handling
- MooTools - DOM Manipulations
- MooTools - Style Properties
- MooTools - Input Filtering
- MooTools - Drag and Drop
- MooTools - Regular Expression
- MooTools - Periodicals
- MooTools - Sliders
- MooTools - Sortables
- MooTools - Accordion
- MooTools - Tooltips
- MooTools - Tabbed Content
- MooTools - Classes
- MooTools - Fx.Element
- MooTools - Fx.Slide
- MooTools - Fx.Tween
- MooTools - Fx.Morph
- MooTools - Fx.Options
- MooTools - Fx.Events
- MooTools Useful Resources
- MooTools - Quick Guide
- MooTools - Useful Resources
- MooTools - Discussion
MooTools - Tooltips
MooTools provides different tooltips to design custom styles and effects. In this chapter, we will learn the various options and events of tooltips, as well as a few tools that will help you add or remove tooltips from elements.
Creating a New Tooltip
Creating a tooltip is very simple. First, we have to create the element where we will attach the tooltip. Let us take an example that creates an anchor tag and adds that to the Tips class in the constructor. Take a look at the following code.
<a id = "tooltipID" class = "tooltip_demo" title = "1st Tooltip Title" rel = "here is the default 'text' for toll tip demo" href = "http://www.tutorialspoint.com">Tool tip _demo</a>
Take a look at the code used to create tooltip.
var customTips = $$('.tooltip_demo'); var toolTips = new Tips(customTips);
Example
The following example explains the basic idea of Tooltips. Take a look at the following code.
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> window.addEvent('domready', function() { var customTips = $$('.tooltip_demo'); var toolTips = new Tips(customTips); }); </script> </head> <body> <a id = "tooltipID" class = "tooltip_demo" title = "1st Tooltip Title" rel = "here is the default 'text' for toll tip demo" href = "http://www.tutorialspoint.com">Tool tip _demo</a> </body> </html>
You will receive the following output −
Output
Tooltip Options
There are only five options in Tips and they are all pretty self-explanatory.
showDelay
An integer measured in milliseconds, this will determine the delay before the tooltip shows once the user mouse onto the element. The default is set at 100.
hideDelay
Just like showDelay above, this integer (also measured in milliseconds) determines how long to wait before hiding the tip once the user leaves the element. The default is set at 100.
className
This lets you set a class name for the tooltip wrap. The default is set to Null.
Offset
This determines how far away from the element the tooltip will appear. ‘x’ refers to the right offset, where ‘y’ is the down offset (both relative to the cursor IF the ‘fixed’ option is set to false, otherwise the offset is relative to the original element). Default is x: 16, y: 16
Fixed
This sets whether or not the tooltip will follow your mouse if you move around the element. If you set it to true, the tooltip will not move when you move your cursor, but will stay fixed relative to the original element. The default is set to false.
Tooltip Events
The tooltip events remain simple, like the rest of this class. There are two events — onShow and onHide, and they work as you would expect.
onShow()
This event executes when the tooltip appears. If you set a delay, this event will not execute until the delay is up.
onHide()
The tooltip hides with the execution of this event. If there is a delay, this event will not execute until the delay is up.
Tooltip Methods
There are two methods for tooltips — attach and detach. This lets you target a specific element and add it to a tooltip object (and thereby, inherent all the settings in that class instance) or detach a particular element.
attach()
To attach a new element to a tooltip object, just state the tip object, the tack on .attach();, and finally place the element selector within the brackets ().
Syntax
toolTips.attach('#tooltipID3');
dettach()
This method works just as the .attach method, but the outcome is completely the opposite. First, state the tip object, then add .dettach(), and finally place your element selector within ().
Syntax
toolTips.dettach('#tooltipID3');
Example
Let us take an example that explains tooltip. Take a look at the following code.
<!DOCTYPE html> <html> <head> <style> .custom_tip .tip { background-color: #333; padding: 5px; } .custom_tip .tip-title { color: #fff; background-color: #666; font-size: 20px; padding: 5px; } .custom_tip .tip-text { color: #fff; padding: 5px; } </style> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> window.addEvent('domready', function() { var customTips = $$('.tooltip_demo'); var toolTips = new Tips(customTips, { showDelay: 1000, //default is 100 hideDelay: 100, //default is 100 className: 'custom_tip', //default is null offsets: { 'x': 100, //default is 16 'y': 16 //default is 16 }, fixed: false, //default is false onShow: function(toolTipElement){ toolTipElement.fade(.8); $('show').highlight('#FFF504'); }, onHide: function(toolTipElement){ toolTipElement.fade(0); $('hide').highlight('#FFF504'); } }); var toolTipsTwo = new Tips('.tooltip2', { className: 'something_else', //default is null }); $('tooltipID1').store('tip:text', 'You can replace the href with whatever text you want.'); $('tooltipID1').store('tip:title', 'Here is a new title.'); $('tooltipID1').set('rel', 'This will not change the tooltips text'); $('tooltipID1').set('title', 'This will not change the tooltips title'); toolTips.detach('#tooltipID2'); toolTips.detach('#tooltipID4'); toolTips.attach('#tooltipID4'); }); </script> </head> <body> <div id = "show" class = "ind">onShow</div> <div id = "hide" class = "ind">onHide</div> <p><a id = "tooltipID1" class = "tooltip_demo" title = "1st Tooltip Title" rel = "here is the default 'text' of 1" href = "http://www.tutorialspoint.com">Tool tip 1</a></p> <p><a id = "tooltipID2" class = "tooltip_demo" title = "2nd Tooltip Title" rel = "here is the default 'text' of 2" href = "http://www.tutorialspoint.com">Tool tip is detached</a></p> <p><a id = "tooltipID3" class = "tooltip_demo_2" title = "3rd Tooltip Title" rel = "here is the default 'text' of 3" href = "http://www.tutorialspoint.com">Tool tip 3</a></p> <p><a id = "tooltipID4" class = "tooltip_demo_2" title = "4th Tooltip Title" rel = "here is the default 'text' of 4, i was detached then attached" href = "http://www.tutorialspoint.com">Tool tip detached then attached again. </a></p> <p><a id = "tooltipID5" class = "tooltip2" title = "Other Tooltip Title" rel = "here is the default 'text' of 'other style'" href = "http://www.tutorialspoint.com/">A differently styled tool tip</a></p> </body> </html>
You will receive the following output −
Output