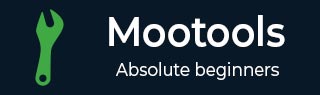
- MooTools Tutorial
- MooTools - Home
- MooTools - Introduction
- MooTools - Installation
- MooTools - Program Structure
- MooTools - Selectors
- MooTools - Using Arrays
- MooTools - Functions
- MooTools - Event Handling
- MooTools - DOM Manipulations
- MooTools - Style Properties
- MooTools - Input Filtering
- MooTools - Drag and Drop
- MooTools - Regular Expression
- MooTools - Periodicals
- MooTools - Sliders
- MooTools - Sortables
- MooTools - Accordion
- MooTools - Tooltips
- MooTools - Tabbed Content
- MooTools - Classes
- MooTools - Fx.Element
- MooTools - Fx.Slide
- MooTools - Fx.Tween
- MooTools - Fx.Morph
- MooTools - Fx.Options
- MooTools - Fx.Events
- MooTools Useful Resources
- MooTools - Quick Guide
- MooTools - Useful Resources
- MooTools - Discussion
MooTools - Regular Expression
MooTools provides a way to create and use Regular Expression (regex). This tutorial will explain the basics and extreme uses of regexes.
Let us discuss a few methods of the regular expressions.
test()
test() is a method used to test the regular expression with the input string. While JavaScript already provides the RegExp object along with the test() function, MooTools adds more features to the RegExp object. Let us take an example and understand how to use the test() method. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> var regex_demo = function(){ var test_string = $('regex_value').get('value'); var regex_value = $('regex_match').get('value'); var test_result = test_string.test(regex_value); if (test_result){ $('regex_1_result').set('html', "Matched"); } else { $('regex_1_result').set('html', "Not Match"); } } window.addEvent('domready', function() { $('regex').addEvent('click', regex_demo); }); </script> </head> <body> String: <input type = "text" id = "regex_value"/><br/><br/> Reg Exp: <input type = "text" id = "regex_match"/><br/><br/> <input type = "button" id = "regex" value = "TEST"/><br/><br/> <Lable id = "regex_1_result"></Lable> </body> </html>
You will receive the following output −
Output
Ignore Case
This is one of the important situations in regular expressions concept. If you don’t want a regular expression to be case sensitive, you call the test method with an option 'I'. Let us take an example that will explain the ignore case in regular expression. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> var regex_demo = function(){ var test_string = $('regex_value').get('value'); var regex_value = $('regex_match').get('value'); var test_result = test_string.test(regex_value, "i"); if (test_result){ $('regex_1_result').set('html', "Matched"); } else { $('regex_1_result').set('html', "Not Match"); } } window.addEvent('domready', function() { $('regex').addEvent('click', regex_demo); }); </script> </head> <body> String: <input type = "text" id = "regex_value"/><br/><br/> Reg Exp: <input type = "text" id = "regex_match"/><br/><br/> <input type = "button" id = "regex" value = "TEST"/><br/><br/> <Lable id = "regex_1_result"></Lable> </body> </html>
You will receive the following output −
Output
Regex starts with '^'
The regex '^' (cap) is a special operator that allows you to check the regular expression in the beginning of a given string. This operator is used as prefix to the regular expression. Let us take an example that will explain how to use this operator. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> var regex_demo = function(){ var test_string = $('regex_value').get('value'); var regex_value = $('regex_match').get('value'); var test_result = test_string.test(regex_value); if (test_result){ $('regex_1_result').set('html', "Matched"); } else { $('regex_1_result').set('html', "Not Match"); } } window.addEvent('domready', function() { $('regex').addEvent('click', regex_demo); }); </script> </head> <body> String: <input type = "text" id = "regex_value"/><br/><br/> Reg Exp: <input type = "text" id = "regex_match"/><br/><br/> <input type = "button" id = "regex" value = "Match"/><br/><br/> <Lable id = "regex_1_result"></Lable> </body> </html>
You will receive the following output −
Output
Regex ends with '$'
The Regex '$' (dollar) is a special operator that allows you to check the regular expression at the end of a given string. This operator is used as suffix to the regular expression. Let us take an example that will explain how to use this operator. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> var regex_demo = function(){ var test_string = $('regex_value').get('value'); var regex_value = $('regex_match').get('value'); var test_result = test_string.test(regex_value); if (test_result){ $('regex_1_result').set('html', "Matched"); } else { $('regex_1_result').set('html', "Not Match"); } } window.addEvent('domready', function() { $('regex').addEvent('click', regex_demo); }); </script> </head> <body> String: <input type = "text" id = "regex_value"/><br/><br/> Reg Exp: <input type = "text" id = "regex_match"/><br/><br/> <input type = "button" id = "regex" value = "Match"/><br/><br/> <Lable id = "regex_1_result"></Lable> </body> </html>
You will receive the following output −
Output
Character Classes
Character classes are a phase of regular expressions that allow you to match specific characters (A or Z) or range of characters (A — Z). For example, you want to test if either of the words foo and zoo exist in a string, classes allow you to do this by placing the characters in the box brackets [] with the regular expressions. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> var regex_demo_1 = function(){ var test_string = $('regex_value').get('value'); var regex_value = $('regex_match_1').get('value'); var test_result = test_string.test(regex_value); if (test_result){ $('regex_1_result').set('html', "Matched"); } else { $('regex_1_result').set('html', "Not Match"); } } var regex_demo_2 = function(){ var test_string = $('regex_value').get('value'); var regex_value = $('regex_match_2').get('value'); var test_result = test_string.test(regex_value); if (test_result){ $('regex_2_result').set('html', "Matched"); } else { $('regex_2_result').set('html', "Not Match"); } } var regex_demo_3 = function(){ var test_string = $('regex_value').get('value'); var regex_value = $('regex_match_3').get('value'); var test_result = test_string.test(regex_value); if (test_result){ $('regex_3_result').set('html', "Matched"); } else { $('regex_3_result').set('html', "Not Match"); } } window.addEvent('domready', function() { $('regex_1').addEvent('click', regex_demo_1); $('regex_2').addEvent('click', regex_demo_2); $('regex_3').addEvent('click', regex_demo_3); }); </script> </head> <body> String: <input type = "text" id = "regex_value"/><br/><br/> Reg Exp 1: <input type = "text" id = "regex_match_1"/> <input type = "button" id = "regex_1" value = "Match"/>  <Lable id = "regex_1_result"></Lable><br/><br/> Reg Exp 2: <input type = "text" id = "regex_match_2"/> <input type = "button" id = "regex_2" value = "Match"/> <Lable id = "regex_2_result"></Lable><br/><br/> Reg Exp 3: <input type = "text" id = "regex_match_3"/> <input type = "button" id = "regex_3" value = "Match"/> <Lable id = "regex_3_result"></Lable> </body> </html>
You will receive the following output −
Output
escapeRegExp()
This method is used to ignore the escape characters from a given string while checking it with a regular expression. Usually, the escape characters are −
- . * + ? ^ $ { } ( ) | [ ] / \
Let us take an example wherein, we have a given String like "[check-this-stuff] it is $900". If you want to take this whole string you have to declare it like this — "\[check\-this\-stuff\] it is \$900". The system accepts only this pattern. We do not use the escakpe character patterns in MooTools. We have the escapeRegExp() method to ignore escape characters. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> var regex_demo_1 = function(){ var test_string = $('regex_value').get('value'); var regex_value = $('regex_match_1').get('value'); var test_result = test_string.test(regex_value); if (test_result){ $('regex_1_result').set('html', "Matched"); } else { $('regex_1_result').set('html', "Not Match"); } } var regex_demo_2 = function(){ var test_string = $('regex_value').get('value'); var regex_value = $('regex_match_1').get('value'); regex_value = regex_value.escapeRegExp(); var test_result = test_string.test(regex_value); if (test_result){ $('regex_2_result').set('html', "Matched"); } else { $('regex_2_result').set('html', "Not Match"); } } window.addEvent('domready', function() { $('regex_1').addEvent('click', regex_demo_1); $('regex_2').addEvent('click', regex_demo_2); $('regex_3').addEvent('click', regex_demo_3); }); </script> </head> <body> String: <input type = "text" id = "regex_value"/><br/><br/> Reg Exp 1: <input type = "text" id = "regex_match_1" size = "6"/><br/><br/> <input type = "button" id = "regex_1" value = "With escapeRegExp()"/> <Lable id = "regex_1_result"></Lable><br/><br/> <input type = "button" id = "regex_2" value = "Without escapeRegExp()"/> <Lable id = "regex_2_result"></Lable><br/><br/> </body> </html>
You will receive the following output −