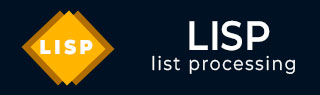
- LISP Tutorial
- LISP - Home
- LISP - Overview
- LISP - Environment
- LISP - Program Structure
- LISP - Basic Syntax
- LISP - Data Types
- LISP - Macros
- LISP - Variables
- LISP - Constants
- LISP - Operators
- LISP - Decisions
- LISP - Loops
- LISP - Functions
- LISP - Predicates
- LISP - Numbers
- LISP - Characters
- LISP - Arrays
- LISP - Strings
- LISP - Sequences
- LISP - Lists
- LISP - Symbols
- LISP - Vectors
- LISP - Set
- LISP - Tree
- LISP - Hash Table
- LISP - Input & Output
- LISP - File I/O
- LISP - Structures
- LISP - Packages
- LISP - Error Handling
- LISP - CLOS
- LISP Useful Resources
- Lisp - Quick Guide
- Lisp - Useful Resources
- Lisp - Discussion
LISP - Symbols
In LISP, a symbol is a name that represents data objects and interestingly it is also a data object.
What makes symbols special is that they have a component called the property list, or plist.
Property Lists
LISP allows you to assign properties to symbols. For example, let us have a 'person' object. We would like this 'person' object to have properties like name, sex, height, weight, address, profession etc. A property is like an attribute name.
A property list is implemented as a list with an even number (possibly zero) of elements. Each pair of elements in the list constitutes an entry; the first item is the indicator, and the second is the value.
When a symbol is created, its property list is initially empty. Properties are created by using get within a setf form.
For example, the following statements allow us to assign properties title, author and publisher, and respective values, to an object named (symbol) 'book'.
Example 1
Create a new source code file named main.lisp and type the following code in it.
(write (setf (get 'books'title) '(Gone with the Wind))) (terpri) (write (setf (get 'books 'author) '(Margaret Michel))) (terpri) (write (setf (get 'books 'publisher) '(Warner Books)))
When you execute the code, it returns the following result −
(GONE WITH THE WIND) (MARGARET MICHEL) (WARNER BOOKS)
Various property list functions allow you to assign properties as well as retrieve, replace or remove the properties of a symbol.
The get function returns the property list of symbol for a given indicator. It has the following syntax −
get symbol indicator &optional default
The get function looks for the property list of the given symbol for the specified indicator, if found then it returns the corresponding value; otherwise default is returned (or nil, if a default value is not specified).
Example 2
Create a new source code file named main.lisp and type the following code in it.
(setf (get 'books 'title) '(Gone with the Wind)) (setf (get 'books 'author) '(Margaret Micheal)) (setf (get 'books 'publisher) '(Warner Books)) (write (get 'books 'title)) (terpri) (write (get 'books 'author)) (terpri) (write (get 'books 'publisher))
When you execute the code, it returns the following result −
(GONE WITH THE WIND) (MARGARET MICHEAL) (WARNER BOOKS)
The symbol-plist function allows you to see all the properties of a symbol.
Example 3
Create a new source code file named main.lisp and type the following code in it.
(setf (get 'annie 'age) 43) (setf (get 'annie 'job) 'accountant) (setf (get 'annie 'sex) 'female) (setf (get 'annie 'children) 3) (terpri) (write (symbol-plist 'annie))
When you execute the code, it returns the following result −
(CHILDREN 3 SEX FEMALE JOB ACCOUNTANT AGE 43)
The remprop function removes the specified property from a symbol.
Example 4
Create a new source code file named main.lisp and type the following code in it.
(setf (get 'annie 'age) 43) (setf (get 'annie 'job) 'accountant) (setf (get 'annie 'sex) 'female) (setf (get 'annie 'children) 3) (terpri) (write (symbol-plist 'annie)) (remprop 'annie 'age) (terpri) (write (symbol-plist 'annie))
When you execute the code, it returns the following result −
(CHILDREN 3 SEX FEMALE JOB ACCOUNTANT AGE 43) (CHILDREN 3 SEX FEMALE JOB ACCOUNTANT)