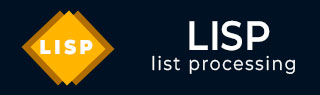
- LISP Tutorial
- LISP - Home
- LISP - Overview
- LISP - Environment
- LISP - Program Structure
- LISP - Basic Syntax
- LISP - Data Types
- LISP - Macros
- LISP - Variables
- LISP - Constants
- LISP - Operators
- LISP - Decisions
- LISP - Loops
- LISP - Functions
- LISP - Predicates
- LISP - Numbers
- LISP - Characters
- LISP - Arrays
- LISP - Strings
- LISP - Sequences
- LISP - Lists
- LISP - Symbols
- LISP - Vectors
- LISP - Set
- LISP - Tree
- LISP - Hash Table
- LISP - Input & Output
- LISP - File I/O
- LISP - Structures
- LISP - Packages
- LISP - Error Handling
- LISP - CLOS
- LISP Useful Resources
- Lisp - Quick Guide
- Lisp - Useful Resources
- Lisp - Discussion
LISP - Bitwise Operators
Bitwise operators work on bits and perform bit-by-bit operation. The truth tables for bitwise and, or, and xor operations are as follows −
p | q | p and q | p or q | p xor q |
---|---|---|---|---|
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
1 | 0 | 0 | 1 | 1 |
Assume if A = 60; and B = 13; now in binary format they will be as follows: A = 0011 1100 B = 0000 1101 ----------------- A and B = 0000 1100 A or B = 0011 1101 A xor B = 0011 0001 not A = 1100 0011
The Bitwise operators supported by LISP are listed in the following table. Assume variable A holds 60 and variable B holds 13, then −
Operator | Description | Example |
---|---|---|
logand | This returns the bit-wise logical AND of its arguments. If no argument is given, then the result is -1, which is an identity for this operation. | (logand a b)) will give 12 |
logior | This returns the bit-wise logical INCLUSIVE OR of its arguments. If no argument is given, then the result is zero, which is an identity for this operation. | (logior a b) will give 61 |
logxor | This returns the bit-wise logical EXCLUSIVE OR of its arguments. If no argument is given, then the result is zero, which is an identity for this operation. | (logxor a b) will give 49 |
lognor | This returns the bit-wise NOT of its arguments. If no argument is given, then the result is -1, which is an identity for this operation. | (lognor a b) will give -62, |
logeqv | This returns the bit-wise logical EQUIVALENCE (also known as exclusive nor) of its arguments. If no argument is given, then the result is -1, which is an identity for this operation. | (logeqv a b) will give -50 |
Example
Create a new source code file named main.lisp and type the following code in it.
(setq a 60) (setq b 13) (format t "~% BITWISE AND of a and b is ~a" (logand a b)) (format t "~% BITWISE INCLUSIVE OR of a and b is ~a" (logior a b)) (format t "~% BITWISE EXCLUSIVE OR of a and b is ~a" (logxor a b)) (format t "~% A NOT B is ~a" (lognor a b)) (format t "~% A EQUIVALANCE B is ~a" (logeqv a b)) (terpri) (terpri) (setq a 10) (setq b 0) (setq c 30) (setq d 40) (format t "~% Result of bitwise and operation on 10, 0, 30, 40 is ~a" (logand a b c d)) (format t "~% Result of bitwise or operation on 10, 0, 30, 40 is ~a" (logior a b c d)) (format t "~% Result of bitwise xor operation on 10, 0, 30, 40 is ~a" (logxor a b c d)) (format t "~% Result of bitwise eqivalance operation on 10, 0, 30, 40 is ~a" (logeqv a b c d))
When you click the Execute button, or type Ctrl+E, LISP executes it immediately and the result returned is −
BITWISE AND of a and b is 12 BITWISE INCLUSIVE OR of a and b is 61 BITWISE EXCLUSIVE OR of a and b is 49 A NOT B is -62 A EQUIVALANCE B is -50 Result of bitwise and operation on 10, 0, 30, 40 is 0 Result of bitwise or operation on 10, 0, 30, 40 is 62 Result of bitwise xor operation on 10, 0, 30, 40 is 60 Result of bitwise eqivalance operation on 10, 0, 30, 40 is -61
lisp_operators.htm
Advertisements