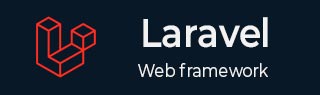
- Laravel Tutorial
- Laravel - Home
- Laravel - Overview
- Laravel - Installation
- Laravel - Application Structure
- Laravel - Configuration
- Laravel - Routing
- Laravel - Middleware
- Laravel - Namespaces
- Laravel - Controllers
- Laravel - Request
- Laravel - Cookie
- Laravel - Response
- Laravel - Views
- Laravel - Blade Templates
- Laravel - Redirections
- Laravel - Working With Database
- Laravel - Errors & Logging
- Laravel - Forms
- Laravel - Localization
- Laravel - Session
- Laravel - Validation
- Laravel - File Uploading
- Laravel - Sending Email
- Laravel - Ajax
- Laravel - Error Handling
- Laravel - Event Handling
- Laravel - Facades
- Laravel - Contracts
- Laravel - CSRF Protection
- Laravel - Authentication
- Laravel - Authorization
- Laravel - Artisan Console
- Laravel - Encryption
- Laravel - Hashing
- Understanding Release Process
- Laravel - Guest User Gates
- Laravel - Artisan Commands
- Laravel - Pagination Customizations
- Laravel - Dump Server
- Laravel - Action URL
- Laravel Useful Resources
- Laravel - Quick Guide
- Laravel - Useful Resources
- Laravel - Discussion
Laravel - Pagination Customizations
Laravel includes a feature of pagination which helps a user or a developer to include a pagination feature. Laravel paginator is integrated with the query builder and Eloquent ORM. The paginate method automatically takes care of setting the required limit and the defined offset. It accepts only one parameter to paginate i.e. the number of items to be displayed in one page.
Laravel 5.7 includes a new pagination method to customize the number of pages on each side of the paginator. The new method no longer needs a custom pagination view.
The custom pagination view code demonstration is mentioned below −
<?php namespace App\Http\Controllers; use Illuminate\Support\Facades\DB; use App\Http\Controllers\Controller; class UserController extends Controller{ /** * Show all of the users for the application. * * @return Response */ public function index() { $users = DB::table('users')->paginate(15); return view('user.index', ['users' => $users]); } }
The new pagination customization as per Laravel standards is mentioned below −
<?php User::paginate(10)->onEachSide(5);
Note that onEachSide refers to the subdivision of each pagination records with 10 and subdivision of 5.