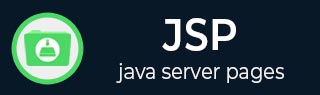
- Basic JSP Tutorial
- JSP - Home
- JSP - Overview
- JSP - Environment Setup
- JSP - Architecture
- JSP - Lifecycle
- JSP - Syntax
- JSP - Directives
- JSP - Actions
- JSP - Implicit Objects
- JSP - Client Request
- JSP - Server Response
- JSP - Http Status Codes
- JSP - Form Processing
- JSP - Writing Filters
- JSP - Cookies Handling
- JSP - Session Tracking
- JSP - File Uploading
- JSP - Handling Date
- JSP - Page Redirect
- JSP - Hits Counter
- JSP - Auto Refresh
- JSP - Sending Email
- Advanced JSP Tutorials
- JSP - Standard Tag Library
- JSP - Database Access
- JSP - XML Data
- JSP - Java Beans
- JSP - Custom Tags
- JSP - Expression Language
- JSP - Exception Handling
- JSP - Debugging
- JSP - Security
- JSP - Internationalization
- JSP Useful Resources
- JSP - Questions and Answers
- JSP - Quick Guide
- JSP - Useful Resources
- JSP - Discussion
JSTL - Core <fmt:formatNumber> Tag
The <fmt:formatNumber> tag is used to format numbers, percentages, and currencies.
Attribute
The <fmt:formatNumber> tag has the following attributes −
Attribute | Description | Required | Default |
---|---|---|---|
Value | Numeric value to display | Yes | None |
type | NUMBER, CURRENCY, or PERCENT | No | Number |
pattern | Specify a custom formatting pattern for the output. | No | None |
currencyCode | Currency code (for type = "currency") | No | From the default locale |
currencySymbol | Currency symbol (for type = "currency") | No | From the default locale |
groupingUsed | Whether to group numbers (TRUE or FALSE) | No | true |
maxIntegerDigits | Maximum number of integer digits to print | No | None |
minIntegerDigits | Minimum number of integer digits to print | No | None |
maxFractionDigits | Maximum number of fractional digits to print | No | None |
minFractionDigits | Minimum number of fractional digits to print | No | None |
var | Name of the variable to store the formatted number | No | Print to page |
scope | Scope of the variable to store the formatted number | No | page |
If the type attribute is percent or number, then you can use several number-formatting attributes. The maxIntegerDigits and minIntegerDigits attributes allow you to specify the size of the nonfractional portion of the number. If the actual number exceeds maxIntegerDigits, then the number is truncated.
Attributes are also provided to allow you to determine how many decimal places should be used. The minFractionalDigits and maxFractionalDigits attributes allow you to specify the number of decimal places. If the number exceeds the maximum number of fractional digits, the number will be rounded.
Grouping can be used to insert commas between thousands groups. Grouping is specified by setting the groupingIsUsed attribute to either true or false. When using grouping with minIntegerDigits, you must be careful to get your intended result.
You may select to use the pattern attribute. This attribute lets you include special characters that specify how you would like your number encoded. Following table lists out the codes.
S.No. | Symbol & Description |
---|---|
1 | 0 Represents a digit. |
2 | E Represents in exponential form. |
3 | # Represents a digit; displays 0 as absent. |
4 | . Serves as a placeholder for a decimal separator. |
5 | , Serves as a placeholder for a grouping separator. |
6 | ; Separates formats. |
7 | - Used as the default negative prefix. |
8 | % Multiplies by 100 and displays as a percentage. |
9 | ? Multiplies by 1000 and displays as per mille. |
10 | ¤ Represents the currency sign; replaced by actional currency symbol. |
11 | X Indicates that any other characters can be used in the prefix or suffix. |
12 | ' Used to quote special characters in a prefix or suffix. |
Example
<%@ taglib prefix = "c" uri = "http://java.sun.com/jsp/jstl/core" %> <%@ taglib prefix = "fmt" uri = "http://java.sun.com/jsp/jstl/fmt" %> <html> <head> <title>JSTL fmt:formatNumber Tag</title> </head> <body> <h3>Number Format:</h3> <c:set var = "balance" value = "120000.2309" /> <p>Formatted Number (1): <fmt:formatNumber value = "${balance}" type = "currency"/></p> <p>Formatted Number (2): <fmt:formatNumber type = "number" maxIntegerDigits = "3" value = "${balance}" /></p> <p>Formatted Number (3): <fmt:formatNumber type = "number" maxFractionDigits = "3" value = "${balance}" /></p> <p>Formatted Number (4): <fmt:formatNumber type = "number" groupingUsed = "false" value = "${balance}" /></p> <p>Formatted Number (5): <fmt:formatNumber type = "percent" maxIntegerDigits="3" value = "${balance}" /></p> <p>Formatted Number (6): <fmt:formatNumber type = "percent" minFractionDigits = "10" value = "${balance}" /></p> <p>Formatted Number (7): <fmt:formatNumber type = "percent" maxIntegerDigits = "3" value = "${balance}" /></p> <p>Formatted Number (8): <fmt:formatNumber type = "number" pattern = "###.###E0" value = "${balance}" /></p> <p>Currency in USA : <fmt:setLocale value = "en_US"/> <fmt:formatNumber value = "${balance}" type = "currency"/> </p> </body> </html>
The above code will generate the following result −
Number Format:
Formatted Number (1): £120,000.23
Formatted Number (2): 000.231
Formatted Number (3): 120,000.231
Formatted Number (4): 120000.231
Formatted Number (5): 023%
Formatted Number (6): 12,000,023.0900000000%
Formatted Number (7): 023%
Formatted Number (8): 120E3
Currency in USA : $120,000.23