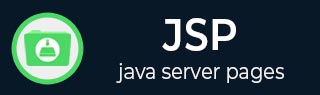
- Basic JSP Tutorial
- JSP - Home
- JSP - Overview
- JSP - Environment Setup
- JSP - Architecture
- JSP - Lifecycle
- JSP - Syntax
- JSP - Directives
- JSP - Actions
- JSP - Implicit Objects
- JSP - Client Request
- JSP - Server Response
- JSP - Http Status Codes
- JSP - Form Processing
- JSP - Writing Filters
- JSP - Cookies Handling
- JSP - Session Tracking
- JSP - File Uploading
- JSP - Handling Date
- JSP - Page Redirect
- JSP - Hits Counter
- JSP - Auto Refresh
- JSP - Sending Email
- Advanced JSP Tutorials
- JSP - Standard Tag Library
- JSP - Database Access
- JSP - XML Data
- JSP - Java Beans
- JSP - Custom Tags
- JSP - Expression Language
- JSP - Exception Handling
- JSP - Debugging
- JSP - Security
- JSP - Internationalization
- JSP Useful Resources
- JSP - Questions and Answers
- JSP - Quick Guide
- JSP - Useful Resources
- JSP - Discussion
JSP - Page Redirecting
In this chapter, we will discuss page redirecting with JSP. Page redirection is generally used when a document moves to a new location and we need to send the client to this new location. This can be because of load balancing, or for simple randomization.
The simplest way of redirecting a request to another page is by using sendRedirect() method of response object. Following is the signature of this method −
public void response.sendRedirect(String location) throws IOException
This method sends back the response to the browser along with the status code and new page location. You can also use the setStatus() and the setHeader() methods together to achieve the same redirection example −
.... String site = "http://www.newpage.com" ; response.setStatus(response.SC_MOVED_TEMPORARILY); response.setHeader("Location", site); ....
Example
This example shows how a JSP performs page redirection to an another location −
<%@ page import = "java.io.*,java.util.*" %> <html> <head> <title>Page Redirection</title> </head> <body> <center> <h1>Page Redirection</h1> </center> <% // New location to be redirected String site = new String("http://www.photofuntoos.com"); response.setStatus(response.SC_MOVED_TEMPORARILY); response.setHeader("Location", site); %> </body> </html>
Let us now put the above code in PageRedirect.jsp and call this JSP using the URL http://localhost:8080/PageRedirect.jsp. This would take you to the given URL http://www.photofuntoos.com.